C Exercises: Check whether a given number is a Kaprekar number or not
C Numbers: Exercise-6 with Solution
Write a program in C to check whether a given number is a Kaprekar number or not.
Test Data
Input a number: 45
Sample Solution:
C Code:
#include <stdio.h>
#include <stdbool.h>
#include <math.h>
// Function to check if a number is a Kaprekar number
bool chkkaprekar(int n) {
if (n == 1)
return true; // If the number is 1, it's considered a Kaprekar number
int sqr_n = n * n; // Square of the number
int ctr_digits = 0; // Counter for the number of digits in the square
// Count the number of digits in the square of the number
while (sqr_n) {
ctr_digits++;
sqr_n /= 10;
}
sqr_n = n * n; // Reset the square of the number
// Loop to check for Kaprekar property
for (int r_digits = 1; r_digits < ctr_digits; r_digits++) {
int eq_parts = pow(10, r_digits); // Calculate the divisor for splitting the square
if (eq_parts == n)
continue; // Skip when the divisor equals the number itself
// Calculate the sum of the split parts of the square
int sum = sqr_n / eq_parts + sqr_n % eq_parts;
if (sum == n)
return true; // If the sum of split parts equals the number, it's a Kaprekar number
}
return false; // If the conditions aren't met, it's not a Kaprekar number
}
// Main function
int main() {
int kpno;
printf("\n\n Check whether a given number is a Kaprekar number: \n");
printf(" -------------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &kpno); // Read an integer from the user and store it in 'kpno'
// Check if the number is a Kaprekar number and print the result
if (chkkaprekar(kpno) == true) {
printf("%d is a Kaprekar number. \n", kpno);
} else {
printf("%d is not a Kaprekar number. \n", kpno);
}
return 0;
}
Sample Output:
Input a number: 45 45 is a Kaprekar number.
Visual Presentation:
Flowchart:
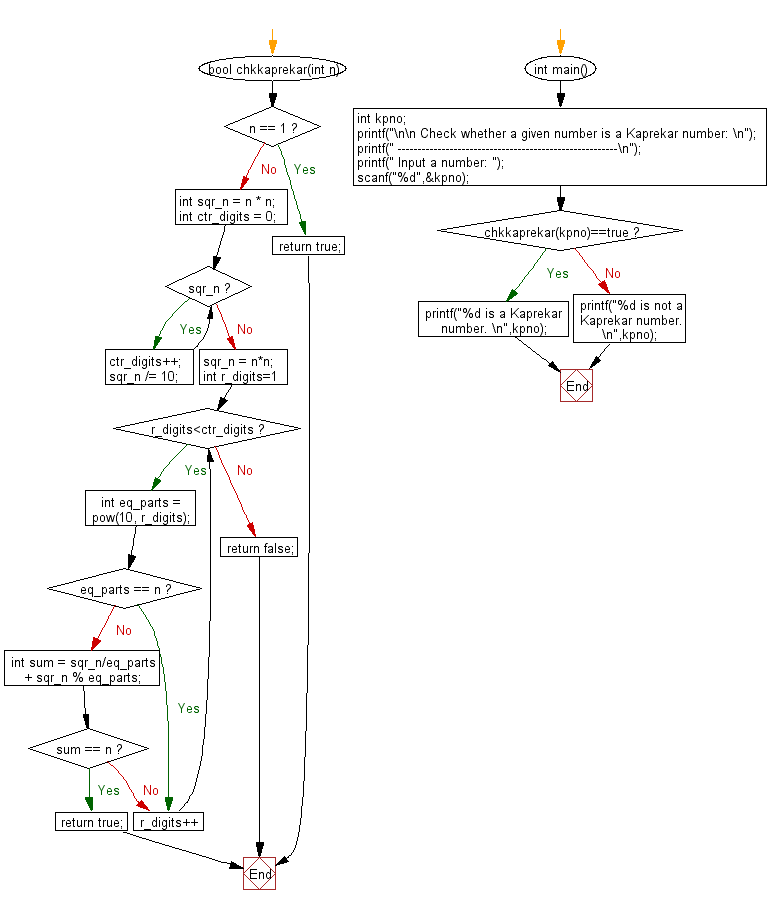
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find the Deficient numbers (integers) between 1 to 100.
Next: Write a program in C to generate and show all Kaprekar numbers less than 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics