C Exercises: Find the Deficient numbers (integers) between 1 to 100
C Numbers: Exercise-5 with Solution
Write a program in C to find the Deficient numbers (integers) between 1 to 100.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdbool.h>
#include <math.h>
// Function to calculate the sum of divisors of a number
int getSum(int n)
{
int sum = 0;
for (int i = 1; i <= sqrt(n); i++) // Loop through numbers from 1 to the square root of 'n'
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
if (n / i == i)
sum = sum + i; // If 'i' is a divisor and is equal to the square root of 'n', add it to 'sum'
else
{
sum = sum + i; // Add 'i' to 'sum'
sum = sum + (n / i); // Add 'n / i' to 'sum'
}
}
}
sum = sum - n; // Subtract the number 'n' from the sum of its divisors
return sum; // Return the sum of divisors
}
// Function to check if a number is a deficient number
bool checkDeficient(int n)
{
return (getSum(n) < n); // Return true if the sum of divisors is less than 'n', otherwise return false
}
// Main function
int main()
{
int n, ctr = 0; // Declare variables 'n' for the number, 'ctr' to count deficient numbers
printf("\n\n The Deficient numbers between 1 to 100 are: \n");
printf(" ------------------------------------------------\n");
for (int j = 1; j <= 100; j++) // Loop through numbers from 1 to 100
{
n = j; // Assign the current value of 'j' to 'n'
if (checkDeficient(n) == true) // Check if 'n' is a deficient number
{
printf("%d ", n); // Print the deficient number
ctr++; // Increment the counter for deficient numbers
}
}
printf("\n The Total number of Deficient numbers are: %d \n", ctr); // Print the total count of deficient numbers
return 0;
}
Sample Output:
The Deficient numbers between 1 to 100 are: ------------------------------------------------ 1 2 3 4 5 7 8 9 10 11 13 14 15 16 17 19 21 22 23 25 26 27...
Visual Presentation:
Flowchart:
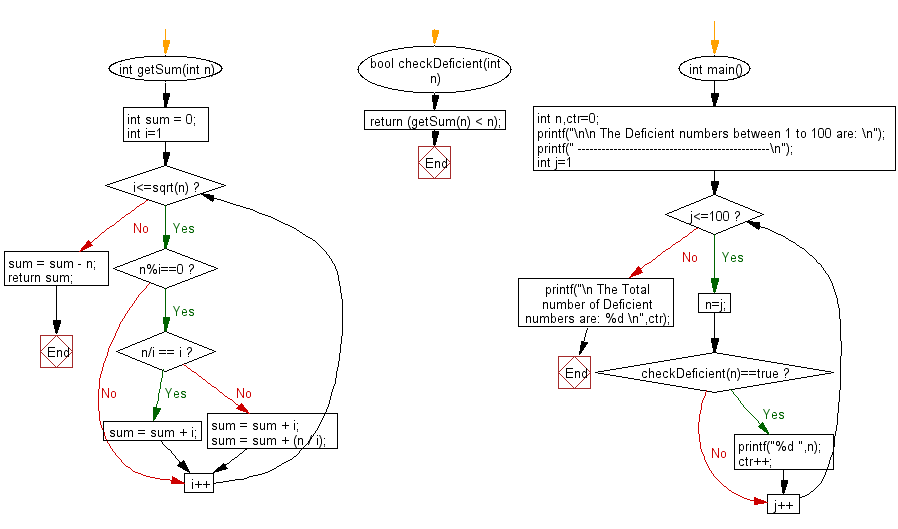
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a given number is Deficient or not.
Next: Write a program in C to check whether a given number is a Kaprekar number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/numbers/c-numbers-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics