C#: Number of letters and digits in a given string
Count Letters and Digits in String
Write a C# Sharp program to get the number of letters and digits in a given string.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declaring a variable to hold text
string text;
// Assigning a value to the 'text' variable
text = "Python 3.0";
// Displaying the original string in the console
Console.WriteLine("Original string:: " + text);
// Calling the 'test' method and displaying the result
Console.WriteLine(test(text));
// Assigning a new value to the 'text' variable
text = "dsfkaso230samdm2423sa";
// Displaying the new original string in the console
Console.WriteLine("\nOriginal string:: " + text);
// Calling the 'test' method again and displaying the result
Console.WriteLine(test(text));
}
// Method to count the number of letters and digits in a string
public static string test(string text)
{
// Counting the number of letters in the given 'text' using LINQ
int ctr_letters = text.Count(char.IsLetter);
// Counting the number of digits in the given 'text' using LINQ
int ctr_digits = text.Count(char.IsDigit);
// Returning the count of letters and digits as a string
return "Number of letters: " + ctr_letters + " Number of digits: " + ctr_digits;
}
}
}
Sample Output:
Original string:: Python 3.0 Number of letters: 6 Number of digits: 2 Original string:: dsfkaso230samdm2423sa Number of letters: 14 Number of digits: 7
Flowchart:
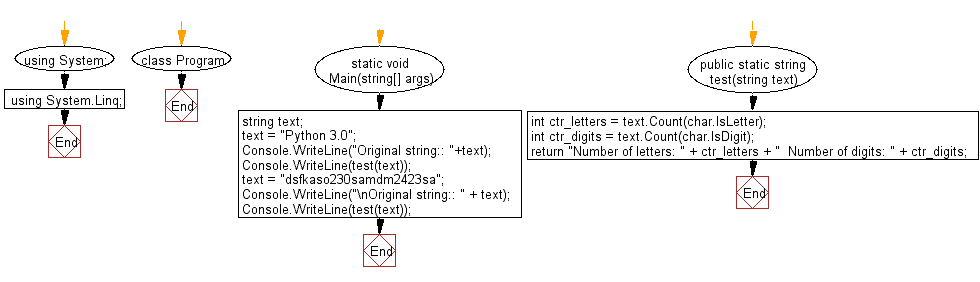
For more Practice: Solve these Related Problems:
- Write a C# program to count uppercase letters, lowercase letters, digits, and special characters in a string.
- Write a C# program to find the total number of alphanumeric characters in a string excluding white spaces.
- Write a C# program to count letters and digits only if they appear in alternating order in a string.
- Write a C# program to compute the ratio of letters to digits in a given alphanumeric string.
Go to:
PREV : Cumulative Sum of Array.
NEXT : Reverse Boolean Value.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.