C#: Reverse a boolean value
Reverse Boolean Value
Write a C# Sharp program to reverse a Boolean value.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declaring and initializing boolean variables
bool cat = false;
bool dog = true;
// Displaying the original value of the 'cat' variable
Console.WriteLine("Original value: " + cat);
// Displaying the reversed value of 'cat' using the 'test' method
Console.WriteLine("Reverse value: " + test(cat));
// Displaying the original value of the 'dog' variable
Console.WriteLine("Original value: " + dog);
// Displaying the reversed value of 'dog' using the 'test' method
Console.WriteLine("Reverse value: " + test(dog));
}
// Method to reverse a boolean value
public static bool test(bool boolean)
{
// Reversing the boolean value using the logical NOT operator (!)
return !boolean;
}
}
}
Sample Output:
Original value: False Reverse value: True Original value: True Reverse value: False
Flowchart:
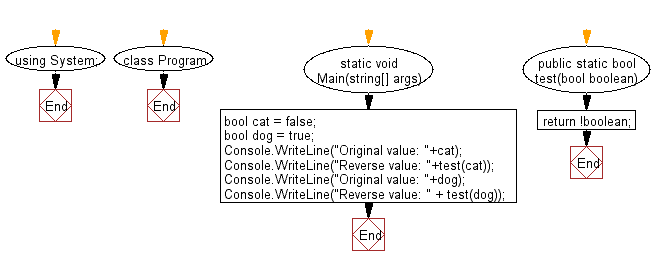
C# Sharp Code Editor:
Previous: Write a C# Sharp program to get the number of letters and digits in a given string.
Next: Write a C# Sharp program to find the sum of the interior angles (in degrees) of a given Polygon. Input number of straight line(s).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.