C#: Determine the HCF of two numbers
C# Sharp For Loop: Exercise-43 with Solution
Write a C# Sharp program to find the HCF (Highest Common Factor) of two numbers.
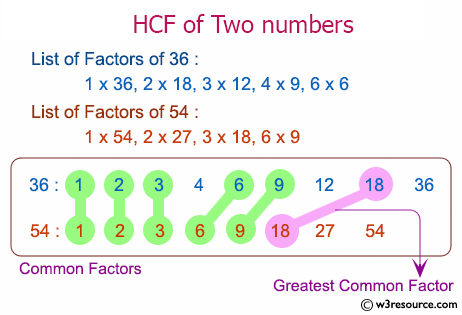
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise43 // Declaration of the Exercise43 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int i, n1, n2, j, hcf = 1;
// Displaying information about determining the HCF of two numbers
Console.Write("\n\n");
Console.Write("Determine the HCF of two numbers:\n");
Console.Write("-----------------------------------");
Console.Write("\n\n");
// Prompting user to input the first number for HCF
Console.Write("Input 1st number for HCF: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Prompting user to input the second number for HCF
Console.Write("Input 2nd number for HCF: ");
n2 = Convert.ToInt32(Console.ReadLine());
j = (n1 < n2) ? n1 : n2; // Determining the smaller number between n1 and n2
// Loop to find the Highest Common Factor (HCF) of n1 and n2
for (i = 1; i <= j; i++)
{
if (n1 % i == 0 && n2 % i == 0)
{
hcf = i; // Updating the HCF whenever both numbers are divisible by 'i'
}
}
// Displaying the calculated HCF of n1 and n2
Console.Write("\nHCF of {0} and {1} is : {2}\n\n", n1, n2, hcf);
}
}
Sample Output:
Determine the HCF of two numbers: ----------------------------------- Input 1st number for HCF: 10 Input 2nd number for HCF: 14 HCF of 10 and 14 is : 2
Flowchart:
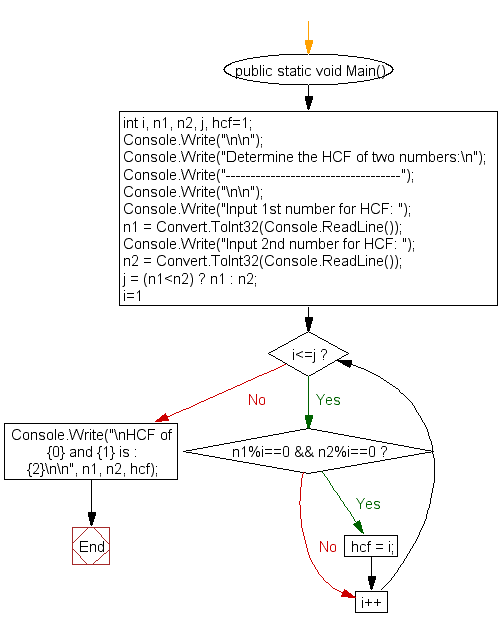
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a binary number into a decimal number without using an array, function and while loop.
Next: Write a program in C# Sharp to find LCM of any two numbers using HCF.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics