C#: Determine the LCM of two numbers using HCF
Write a program in C# Sharp to find the LCM of any two numbers using HCF.
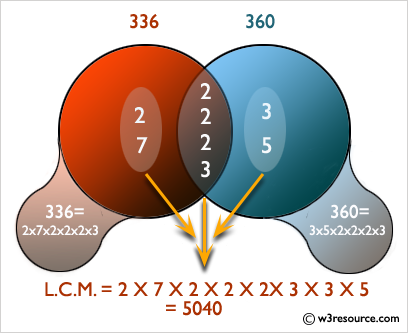
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise44 // Declaration of the Exercise44 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int i, n1, n2, j, hcf = 1, lcm;
// Displaying information about determining the LCM of two numbers using HCF
Console.Write("\n\n");
Console.Write("Determine the LCM of two numbers using HCF:\n");
Console.Write("---------------------------------------------");
Console.Write("\n\n");
// Prompting user to input the first number for LCM
Console.Write("Input 1st number for LCM: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Prompting user to input the second number for LCM
Console.Write("Input 2nd number for LCM: ");
n2 = Convert.ToInt32(Console.ReadLine());
j = (n1 < n2) ? n1 : n2; // Determining the smaller number between n1 and n2
// Loop to find the Highest Common Factor (HCF) of n1 and n2
for (i = 1; i <= j; i++)
{
if (n1 % i == 0 && n2 % i == 0)
{
hcf = i; // Updating the HCF whenever both numbers are divisible by 'i'
}
}
// Calculating the LCM using the formula: (n1 * n2) / HCF
lcm = (n1 * n2) / hcf;
// Displaying the calculated LCM of the given numbers
Console.Write("\nThe LCM of {0} and {1} is : {2}\n\n", n1, n2, lcm);
}
}
Sample Output:
Determine the LCM of two numbers using HCF: --------------------------------------------- Input 1st number for LCM: 8 Input 2nd number for LCM: 12 The LCM of 8 and 12 is : 24
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find HCF (Highest Common Factor) of two numbers.
Next: Write a program in C# Sharp to find LCM of any two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.