C#: Determine the LCM of two numbers
Write a program in C# Sharp to find the LCM of any two numbers.
Visual Presentation:
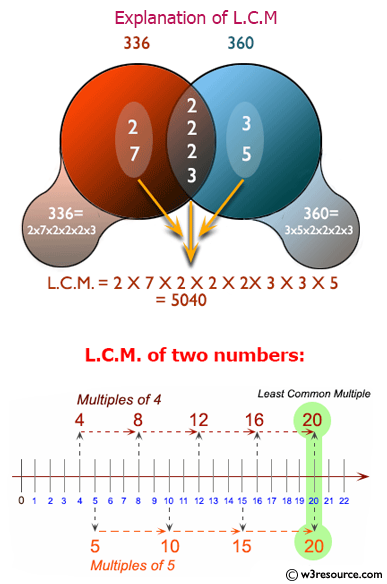
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise45 // Declaration of the Exercise45 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int i, n1, n2, max, lcm = 1;
// Displaying information about determining the LCM of two numbers
Console.Write("\n\n");
Console.Write("Determine the LCM of two numbers:\n");
Console.Write("-----------------------------------");
Console.Write("\n\n");
// Prompting user to input the first number for LCM
Console.Write("Input 1st number for LCM: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Prompting user to input the second number for LCM
Console.Write("Input 2nd number for LCM: ");
n2 = Convert.ToInt32(Console.ReadLine());
max = (n1 > n2) ? n1 : n2; // Determining the greater number between n1 and n2
// Loop to find the LCM using the brute-force approach
for (i = max;; i += max)
{
if (i % n1 == 0 && i % n2 == 0) // Checking if i is divisible by both n1 and n2
{
lcm = i; // Updating the LCM when i is found to be divisible by both
break; // Exiting the loop when the LCM is found
}
}
// Displaying the calculated LCM of the given numbers
Console.Write("\nLCM of {0} and {1} = {2}\n\n", n1, n2, lcm);
}
}
Sample Output:
Determine the LCM of two numbers: ----------------------------------- Input 1st number for LCM: 6 Input 2nd number for LCM: 8 LCM of 6 and 8 = 24
Flowchart:
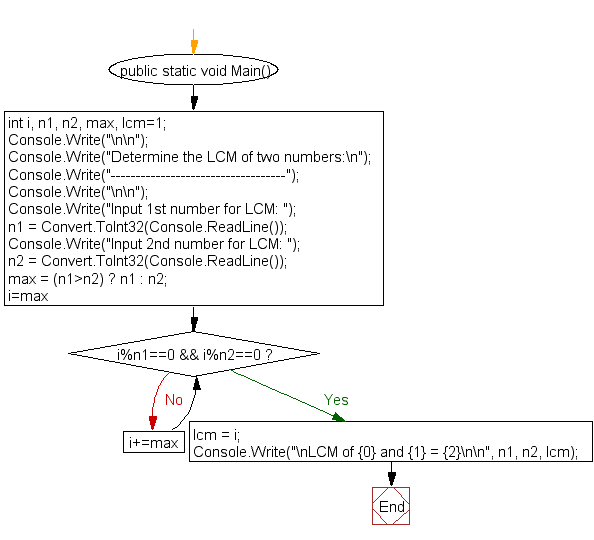
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find LCM of any two numbers using HCF.
Next: Write a program in C# Sharp to convert a binary number into a decimal number using math function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.