C#: Convert a binary number into a decimal using math function
Write a C# Sharp program to convert a binary number into a decimal number using the math function.
Visual Presentation:
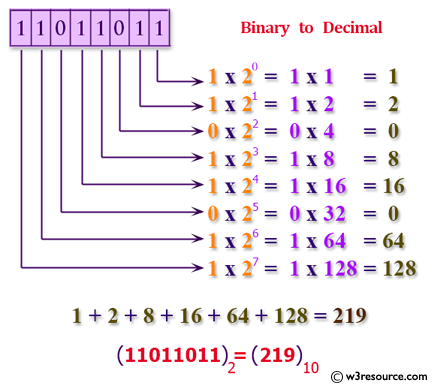
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise46 // Declaration of the Exercise46 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int n1, n;
double dec = 0, i = 0, d;
// Displaying information about converting a binary number into decimal using math function
Console.Write("\n\n");
Console.Write("Convert a binary number into decimal using math function:\n");
Console.Write("-----------------------------------------------------------");
Console.Write("\n\n");
// Prompting user to input the binary number
Console.Write("Input the binary number: ");
n = Convert.ToInt32(Console.ReadLine());
n1 = n; // Storing the original binary number in another variable for display
// Converting binary to decimal
while (n != 0)
{
d = n % 10; // Extracting each digit of the binary number
dec = dec + d * Math.Pow(2, i); // Converting each digit to its decimal equivalent
n = n / 10; // Removing the last digit from the binary number
i++; // Incrementing the power of 2
}
// Displaying the original binary number and its equivalent decimal number
Console.Write("\nThe Binary Number: {0}\nThe equivalent Decimal Number is: {1}\n\n", n1, dec);
}
}
Sample Output:
Convert a binary number into decimal using math function: ----------------------------------------------------------- Input the binary number :1000001 The Binary Number : 1000001 The equivalent Decimal Number is : 65
Flowchart:
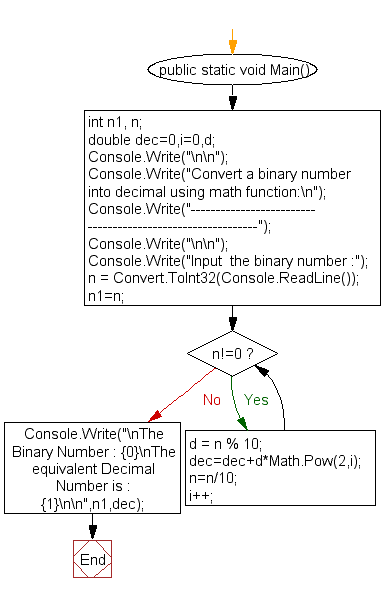
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find LCM of any two numbers.
Next: Write a C# Sharp program to check whether a number is Strong Number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.