C#: Check whether a number is Strong Number or not
Write a C# Sharp program to check whether a number is Strong Number or not.
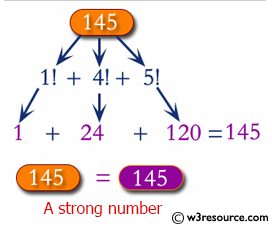
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise47 // Declaration of the Exercise47 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int i, n, n1, s1 = 0, j;
int fact;
// Displaying information about checking whether a number is a Strong number or not
Console.Write("\n\n");
Console.Write("Check whether a number is Strong Number or not:\n");
Console.Write("-------------------------------------------------");
Console.Write("\n\n");
// Prompting user to input a number to check for Strong number
Console.Write("Input a number to check whether it is a Strong number: ");
n = Convert.ToInt32(Console.ReadLine()); // Reading user input
n1 = n; // Storing the original number for comparison
// Loop to calculate the factorial sum of individual digits of the number
for (j = n; j > 0; j = j / 10)
{
fact = 1; // Initializing the factorial as 1 for each new digit
// Loop to calculate the factorial of each digit
for (i = 1; i <= j % 10; i++)
{
fact = fact * i; // Calculating factorial
}
s1 = s1 + fact; // Accumulating the factorial sum
}
// Checking if the sum of factorial of digits is equal to the original number
if (s1 == n1)
{
Console.Write("\n{0} is a Strong number.\n\n", n1); // Displaying result if the number is a Strong number
}
else
{
Console.Write("\n{0} is not a Strong number.\n\n", n1); // Displaying result if the number is not a Strong number
}
}
}
Sample Output:
Check whether a number is Strong Number or not: ------------------------------------------------- Input a number to check whether it is Strong number: 2 2 is Strong number.
Flowchart:
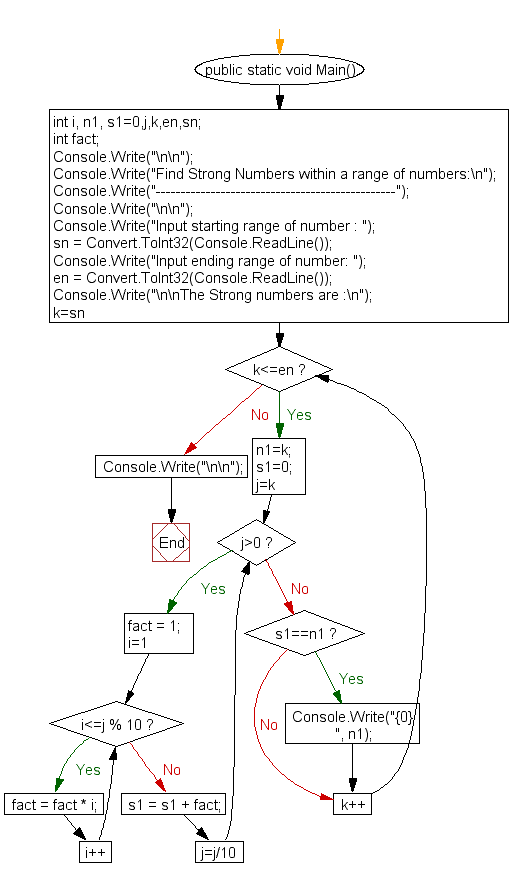
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a binary number into a decimal number using math function.
Next: Write a C# Sharp program to find Strong Numbers within a range of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.