C#: Find Strong Numbers within a range of numbers
Write a C# Sharp program to find Strong Numbers within a range of numbers.
Visual Presentation:
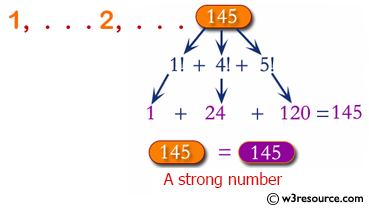
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise48 // Declaration of the Exercise48 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int i, n1, s1 = 0, j, k, en, sn;
int fact;
// Displaying information about finding Strong Numbers within a range
Console.Write("\n\n");
Console.Write("Find Strong Numbers within a range of numbers:\n");
Console.Write("------------------------------------------------");
Console.Write("\n\n");
// Prompting user to input the starting and ending range of numbers
Console.Write("Input starting range of numbers: ");
sn = Convert.ToInt32(Console.ReadLine()); // Reading starting range
Console.Write("Input ending range of numbers: ");
en = Convert.ToInt32(Console.ReadLine()); // Reading ending range
Console.Write("\n\nThe Strong numbers are :\n"); // Displaying the heading
// Loop to find and display the Strong numbers within the specified range
for (k = sn; k <= en; k++)
{
n1 = k; // Storing the original number for comparison
s1 = 0; // Initializing sum of factorials
// Loop to calculate the factorial sum of individual digits of the number
for (j = k; j > 0; j = j / 10)
{
fact = 1; // Initializing factorial as 1 for each new digit
// Loop to calculate the factorial of each digit
for (i = 1; i <= j % 10; i++)
{
fact = fact * i; // Calculating factorial
}
s1 = s1 + fact; // Accumulating the factorial sum
}
// Checking if the sum of factorial of digits is equal to the original number
if (s1 == n1)
{
Console.Write("{0} ", n1); // Displaying Strong number
}
}
Console.Write("\n\n"); // Adding newline after displaying Strong numbers
}
}
Sample Output:
Find Strong Numbers within a range of numbers: ------------------------------------------------ Input starting range of number : 0 Input ending range of number: 1000 The Strong numbers are : 0 1 2 145
Flowchart:
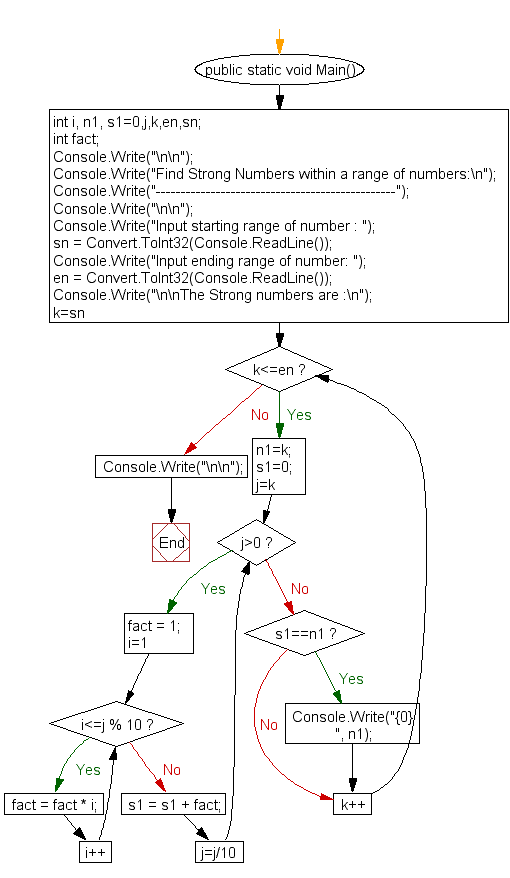
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a number is Strong Number or not.
Next: Write a C# Sharp program to find out the sum of in A.P. series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.