C#: Find out the sum of in A.P. series
C# Sharp For Loop: Exercise-49 with Solution
Write a C# Sharp program to find out the sum of the Arithmetic Progress series.
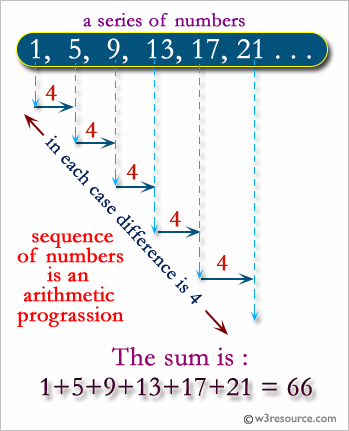
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise49 // Declaration of the Exercise49 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int n1, df, n2, i, ln;
int s1 = 0;
// Displaying information about finding the sum of an A.P. series
Console.Write("\n\n");
Console.Write("Find out the sum of an A.P. series:\n");
Console.Write("-------------------------------------");
Console.Write("\n\n");
// Prompting user to input the starting number, number of items, and common difference
Console.Write("Input the starting number of the A.P. series: ");
n1 = Convert.ToInt32(Console.ReadLine()); // Reading starting number
Console.Write("Input the number of items for the A.P. series: ");
n2 = Convert.ToInt32(Console.ReadLine()); // Reading number of items
Console.Write("Input the common difference of the A.P. series: ");
df = Convert.ToInt32(Console.ReadLine()); // Reading common difference
// Calculating the sum of the arithmetic progression series using the formula
s1 = (n2 * (2 * n1 + (n2 - 1) * df)) / 2;
ln = n1 + (n2 - 1) * df; // Calculating the last number in the series
Console.Write("\nThe Sum of the A.P. series is: \n"); // Displaying the result
// Loop to display the elements of the arithmetic progression series
for (i = n1; i <= ln; i = i + df)
{
// Displaying elements of the series with proper formatting
if (i != ln)
Console.Write("{0} + ", i);
else
Console.Write("{0} = {1} \n\n", i, s1); // Displaying the last element and the sum
}
}
}
Sample Output:
Find out the sum of in A.P. series: ------------------------------------- Input the starting number of the A.P. series: 0 Input the number of items for the A.P. series: 10 Input the common difference of A.P. series: 5 The Sum of the A.P. series are : 0 + 5 + 10 + 15 + 20 + 25 + 30 + 35 + 40 + 45 = 225
Flowchart:
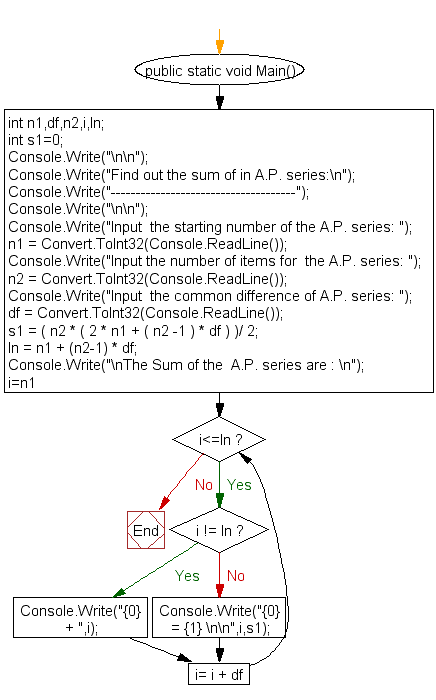
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find Strong Numbers within a range of numbers.
Next: Write a program in C# Sharp to convert a decimal number into octal without an using an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics