C#: Convert decimal number to octal without using an array
Write a program in C# Sharp to convert a decimal number into octal without an using an array.
Visual Presentation:
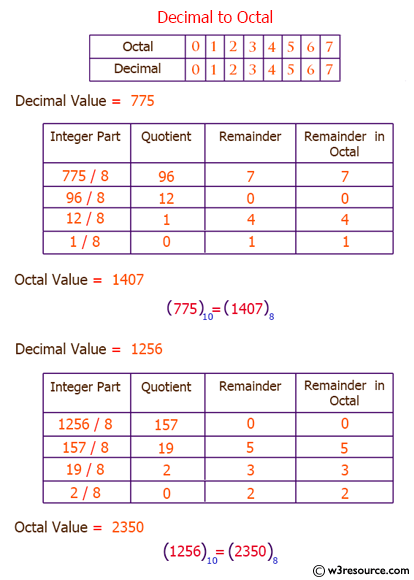
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise50 // Declaration of the Exercise50 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int n, i, j, octalNumber = 0, dn;
// Displaying information about converting decimal to octal
Console.Write("\n\n");
Console.Write("Convert decimal number to octal without using array:\n");
Console.Write("------------------------------------------------------");
Console.Write("\n\n");
// Prompting the user to enter a decimal number
Console.Write("Enter a number to convert : ");
n = Convert.ToInt32(Console.ReadLine()); // Reading the decimal number
dn = n; // Storing the original number for display
i = 1; // Initializing a multiplier for place values in octal system
// Loop to convert decimal to octal without using an array
for (j = n; j > 0; j = j / 8)
{
// Calculating the octal number digit by digit
octalNumber = octalNumber + (j % 8) * i;
i = i * 10; // Incrementing the place value multiplier for next digit
n = n / 8; // Updating the original number
}
// Displaying the converted octal number
Console.Write("\nThe Octal of {0} is {1}.\n\n", dn, octalNumber);
}
}
Sample Output:
Convert decimal number to octal without using array: ------------------------------------------------------ Enter a number to convert : 6 The Octal of 6 is 6.
Flowchart:
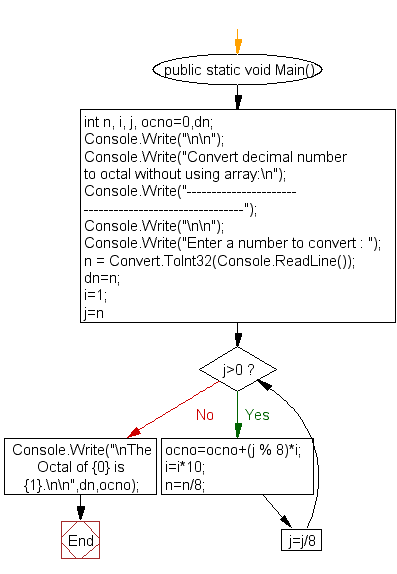
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find out the sum of in A.P. series.
Next: Write a program in C# Sharp to convert a Octal number to Decimal number without using an array, function and while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.