C#: Calculate the sum of the elements in an array
C# Sharp Function: Exercise-5 with Solution
Write a program in C# Sharp to calculate the sum of elements in an array.
Visual Presentation:
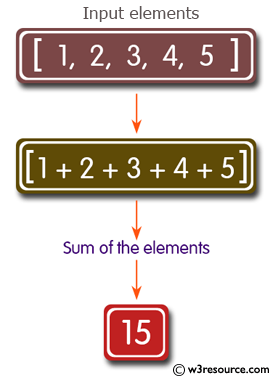
Sample Solution:
C# Sharp Code:
using System;
// Define a public class named 'funcexer5'
public class funcexer5
{
// Define a public static method 'Sum' to calculate the sum of elements in an array
public static int Sum(int[] arr1)
{
// Initialize a variable to store the total sum
int tot = 0;
// Loop through each element in the array
for (int i = 0; i < arr1.Length; i++)
{
// Add each element to the total sum
tot += arr1[i];
}
return tot; // Return the total sum of array elements
}
// Main method, the entry point of the program
public static void Main()
{
// Initialize an array of integers with a length of 5
int[] arr1 = new int[5];
// Print a description of the program
Console.Write("\n\nFunction : Calculate the sum of the elements in an array :\n");
Console.Write("--------------------------------------------------------------\n");
// Prompt the user to input 5 elements into the array
Console.Write("Input 5 elements in the array :\n");
for (int j = 0; j < 5; j++)
{
Console.Write("element - {0} : ", j);
arr1[j] = Convert.ToInt32(Console.ReadLine());
}
// Call the 'Sum' method and display the sum of elements in the array
Console.WriteLine("The sum of the elements of the array is {0}", Sum(arr1));
}
}
Sample Output:
Function : Calculate the sum of the elements in an array : -------------------------------------------------------------- Input 5 elements in the array : element - 0 : 1 element - 1 : 2 element - 2 : 3 element - 3 : 4 element - 4 : 5 The sum of the elements of the array is 15
Flowchart:
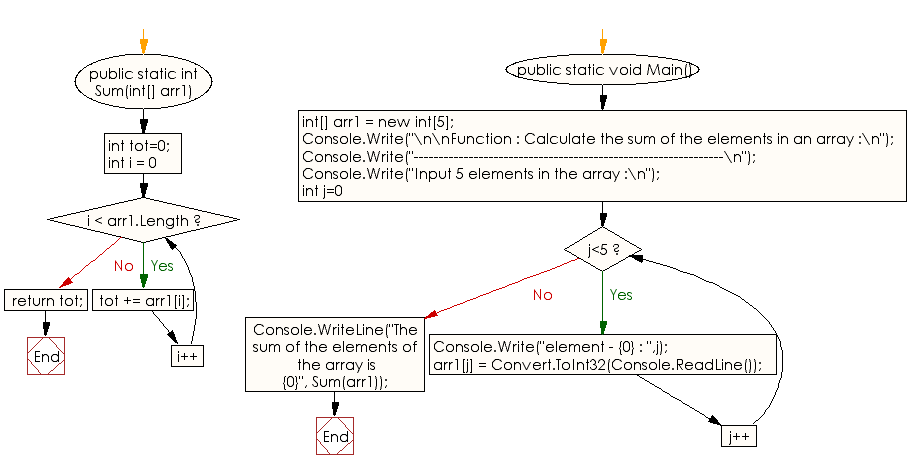
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to input a string and count number of spaces are in the string.
Next: Write a program in C# Sharp to create a function to swap the values of two integer numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics