C# Sharp Exercises: Function : To swap the values of two integer numbers
Write a program in C# Sharp to create a function to swap the values of two integer numbers.
Visual Presentation:
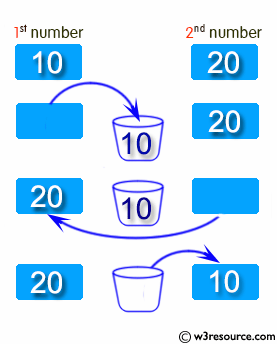
Sample Solution:
C# Sharp Code:
using System;
// Define a public class named 'funcexer6'
public class funcexer6
{
// Define a public static method 'interchange' to swap the values of two integers
public static void interchange(ref int num1, ref int num2)
{
int newnum;
// Store the value of 'num1' in a temporary variable 'newnum'
newnum = num1;
// Assign the value of 'num2' to 'num1'
num1 = num2;
// Assign the value stored in 'newnum' (initially 'num1') to 'num2'
num2 = newnum;
}
// Main method, the entry point of the program
public static void Main()
{
int n1, n2;
// Display a description of the program
Console.Write("\n\nFunction : To swap the values of two integer numbers :\n");
Console.Write("----------------------------------------------------------\n");
// Prompt the user to enter the first number
Console.Write("Enter a number: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Prompt the user to enter the second number
Console.Write("Enter another number: ");
n2 = Convert.ToInt32(Console.ReadLine());
// Call the 'interchange' method to swap the values of 'n1' and 'n2'
interchange(ref n1, ref n2);
// Display the swapped values of 'n1' and 'n2'
Console.WriteLine("Now the 1st number is : {0} , and the 2nd number is : {1}", n1, n2);
}
}
Sample Output:
Function : To swap the values of two integer numbers : ---------------------------------------------------------- Enter a number: 45 Enter another number: 65 Now the 1st number is : 65 , and the 2nd number is : 45
Flowchart :
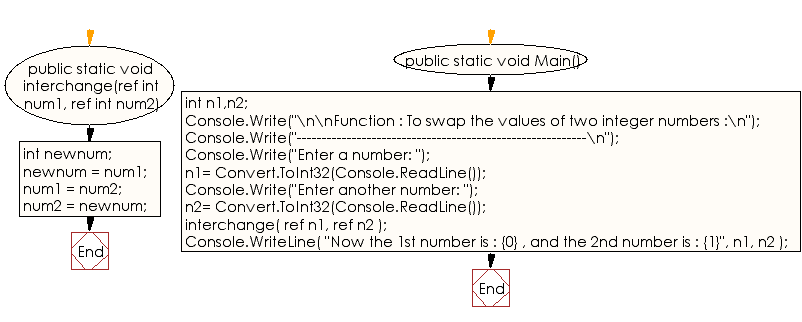
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to calculate the sum of elements in an array.
Next: Write a program in C# Sharp to create a function to calculate the result of raising an integer number to another.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.