C#: Convert a decimal number to binary
C# Sharp Recursion: Exercise-13 with Solution
Write a program in C# Sharp to convert a decimal number to binary using recursion.
Visual Presentation:
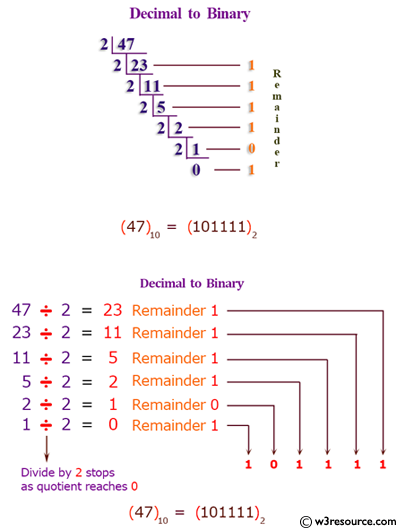
Sample Solution:
C# Sharp Code:
using System;
// Class RecExercise13 to convert a decimal number to binary
class RecExercise13
{
// Main method to execute the program
public static void Main(string[] args)
{
int num;
DecToBinClass pg = new DecToBinClass();
Console.WriteLine("\n\n Recursion : Convert a decimal number to binary :");
Console.WriteLine("------------------------------------------------------");
Console.Write(" Input a decimal number : ");
num = int.Parse(Console.ReadLine());
Console.Write(" The binary equivalent of {0} is : ", num);
pg.deciToBinary(num);
Console.ReadLine();
Console.Write("\n");
}
}
// Class DecToBinClass to perform the decimal to binary conversion
public class DecToBinClass
{
// Method to convert a decimal number to binary recursively
public int deciToBinary(int num)
{
int bin;
if (num != 0)
{
// Calculate binary equivalent using recursion
bin = (num % 2) + 10 * deciToBinary(num / 2);
Console.Write(bin); // Output the binary digit
return 0;
}
else
{
return 0;
}
}
}
Sample Output:
Recursion : Convert a decimal number to binary : ------------------------------------------------------ Input a decimal number : 65 The binary equivalent of 65 is : 1000001
Flowchart :
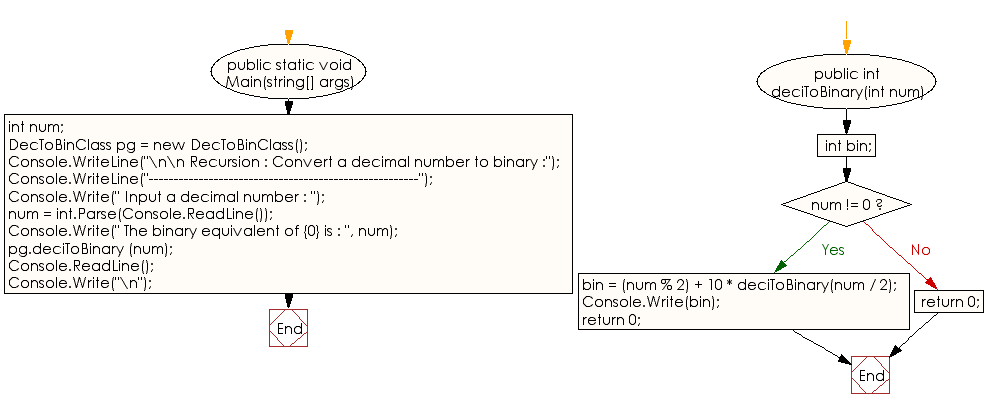
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to find the LCM and GCD of two numbers using recursion.
Next: Write a program in C# Sharp to get the reverse of a string using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics