C#: Get the reverse of a string
C# Sharp Recursion: Exercise-14 with Solution
Write a program in C# Sharp to get the reverse of a string using recursion.
Visual Presentation:
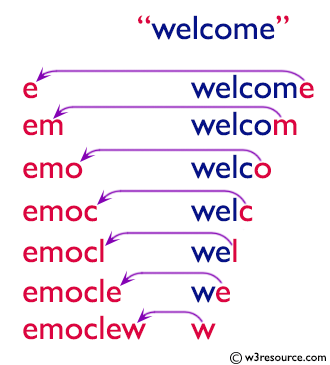
Sample Solution:
C# Sharp Code:
using System;
class RecExercise14
{
static void Main()
{
string str;
// Prompt the user to enter a string
Console.WriteLine("\n\n Recursion : Get the reverse of a string :");
Console.WriteLine("----------------------------------------------");
Console.Write(" Input the string : ");
str = Console.ReadLine();
// Call the StringReverse function to reverse the input string
str = StringReverse(str);
// Display the reversed string
Console.Write(" The reverse of the string is : ");
Console.Write(str);
Console.ReadKey(); // Wait for a key press
Console.Write("\n");
}
// Recursive function to reverse a string
public static string StringReverse(string str)
{
// Check if the string has characters
if (str.Length > 0)
// Reverse the string by taking the last character and calling StringReverse on the substring
return str[str.Length - 1] + StringReverse(str.Substring(0, str.Length - 1));
else
return str; // Return the string if it's empty
}
}
Sample Output:
Recursion : Get the reverse of a string : ---------------------------------------------- Input the string : W3resource The reverse of the string is : ecruoser3W
Flowchart :
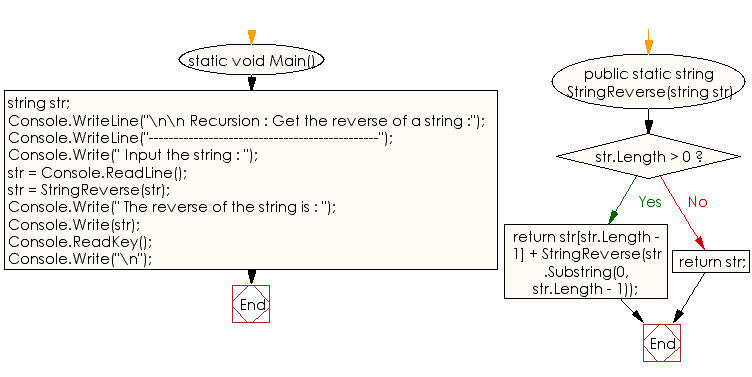
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to convert a decimal number to binary using recursion.
Next: Write a program in C# Sharp to calculate the power of any number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/recursion/csharp-recursion-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics