C#: Extract a substring from a given string
C# Sharp String: Exercise-13 with Solution
Write a program in C# Sharp to extract a substring from a given string without using the library function.
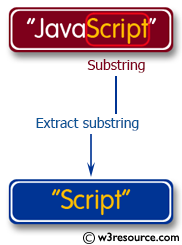
Sample Solution:-
C# Sharp Code:
using System;
// Define the exercise13 class
public class exercise13
{
// Main method - entry point of the program
public static void Main()
{
string str; // Declare a string variable to store input
char[] arr1; // Declare a character array
int pos, l, ln, c = 0; // Declare variables for position, length, string length, and counter
// Prompt the user for input
Console.Write("\n\nExtract a substring from a given string:\n");
Console.Write("--------------------------------------------\n");
Console.Write("Input the string : ");
str = Console.ReadLine(); // Read the input string from the user
ln = str.Length; // Get the length of the input string
arr1 = str.ToCharArray(0, ln); // Convert the input string to a character array
Console.Write("Input the position to start extraction :");
pos = Convert.ToInt32(Console.ReadLine()); // Read the starting position for substring extraction
Console.Write("Input the length of substring :");
l = Convert.ToInt32(Console.ReadLine()); // Read the length of the substring to be extracted
// Output the extracted substring
Console.Write("The substring retrieved from the string is : ");
while (c < l)
{
Console.Write(arr1[pos + c - 1]); // Output each character of the substring
c++;
}
Console.Write("\n\n");
}
}
Sample Output:
Extract a substring from a given string: -------------------------------------------- Input the string : Welcome to w3resource.com Input the position to start extraction :11 Input the length of substring :11 The substring retrieve from the string is : w3resource
Flowchart:
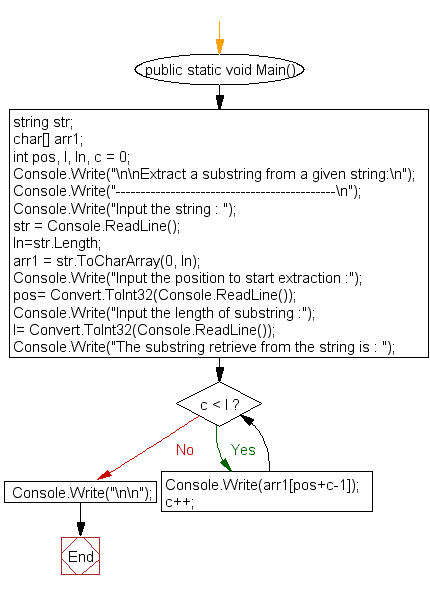
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read a string through the keyboard and sort it using bubble sort.
Next: Write a C# Sharp program to check whether a given substring is present in the given string
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics