C#: Sorts the strings of an array using bubble sort
Write a C# Sharp program to read a string through the keyboard and sort it using bubble sort.
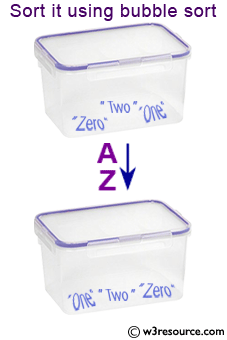
Sample Solution:-
C# Sharp Code:
using System;
// Define the exercise12 class
public class exercise12
{
// Main method - entry point of the program
public static void Main()
{
string[] arr1; // Declare an array to store strings
string temp; // Declare a temporary string variable
int n, i, j, l; // Declare variables for number of strings, iteration, and string length
// Prompt the user to input the number of strings
Console.Write("\n\nSorts the strings of an array using bubble sort :\n");
Console.Write("-----------------------------------------------------\n");
Console.Write("Input number of strings :");
n = Convert.ToInt32(Console.ReadLine()); // Read the number of strings from user input
arr1 = new string[n]; // Initialize the string array with the given number of strings
Console.Write("Input {0} strings below :\n", n);
// Read the strings from the user input
for (i = 0; i < n; i++)
{
arr1[i] = Console.ReadLine(); // Store each string in the array
}
l = arr1.Length; // Get the length of the string array
// Bubble sort algorithm to sort the array of strings
for (i = 0; i < l; i++)
{
for (j = 0; j < l - 1; j++)
{
// Compare adjacent strings and swap them if they are in the wrong order
if (arr1[j].CompareTo(arr1[j + 1]) > 0)
{
temp = arr1[j];
arr1[j] = arr1[j + 1];
arr1[j + 1] = temp;
}
}
}
// Display the sorted array of strings
Console.Write("\n\nAfter sorting the array appears like : \n");
for (i = 0; i < l; i++)
{
Console.WriteLine(arr1[i] + " "); // Print each string in the sorted array
}
}
}
Sample Output:
Sorts the strings of an array using bubble sort : ----------------------------------------------------- Input number of strings :3 Input 3 strings below : IJKL EFGH ABCD After sorting the array appears like : ABCD EFGH IJKL
Flowchart:
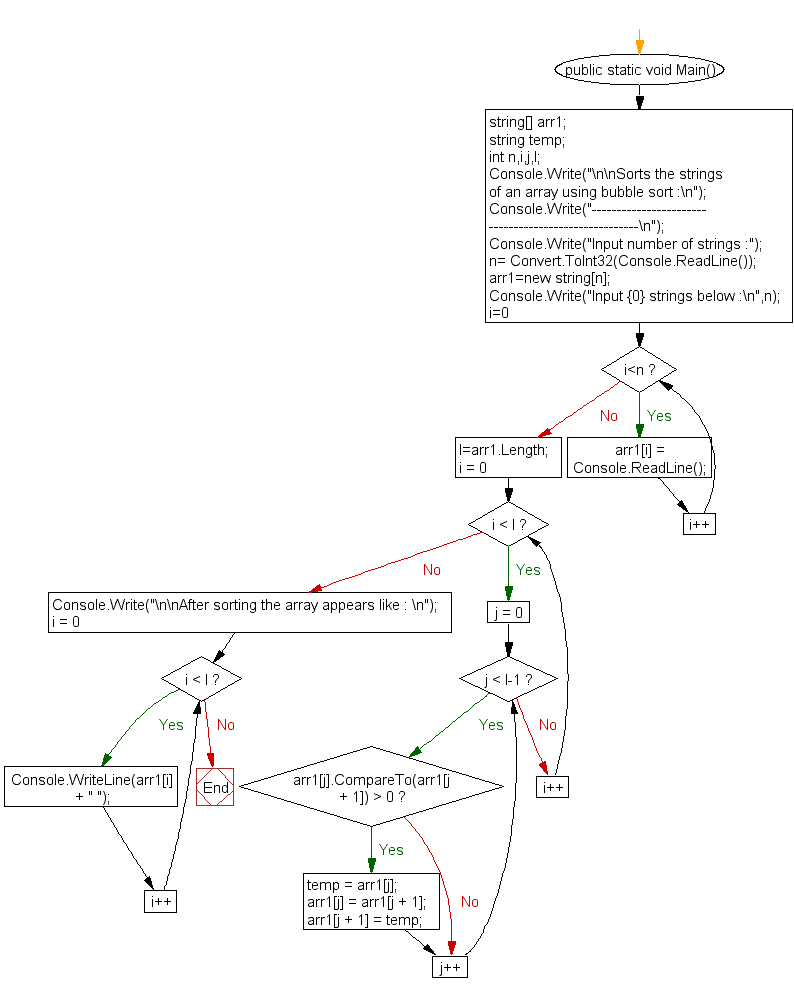
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to sort a string array in ascending order.
Next: Write a program in C# Sharp to extract a substring from a given string without using the library function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.