Java: Get the difference between the largest and smallest values in an array of integers
Java Array: Exercise-28 with Solution
Write a Java program to get the difference between the largest and smallest values in an array of integers. The array must have a length of at least 1.
Pictorial Presentation:
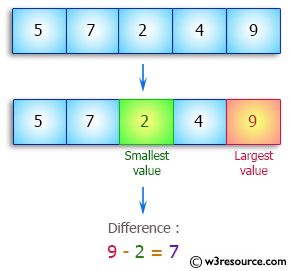
Sample Solution:
Java Code:
// Import the java.util package to use utility classes, including Arrays.
import java.util.Arrays;
// Define a class named Exercise28.
public class Exercise28 {
// The main method for executing the program.
public static void main(String[] args) {
// Declare and initialize an array of integers.
int[] array_nums = {5, 7, 2, 4, 9};
// Print the original array.
System.out.println("Original Array: " + Arrays.toString(array_nums));
// Initialize variables to store the maximum and minimum values.
int max_val = array_nums[0];
int min = array_nums[0];
// Use a loop to find the maximum and minimum values in the array.
for (int i = 1; i < array_nums.length; i++) {
if (array_nums[i] > max_val)
max_val = array_nums[i];
else if (array_nums[i] < min)
min = array_nums[i];
}
// Calculate and print the difference between the largest and smallest values.
System.out.println("Difference between the largest and smallest values of the said array: " + (max_val - min));
}
}
Sample Output:
Original Array: [5, 7, 2, 4, 9] Difference between the largest and smallest values of the said array: 7
Flowchart:
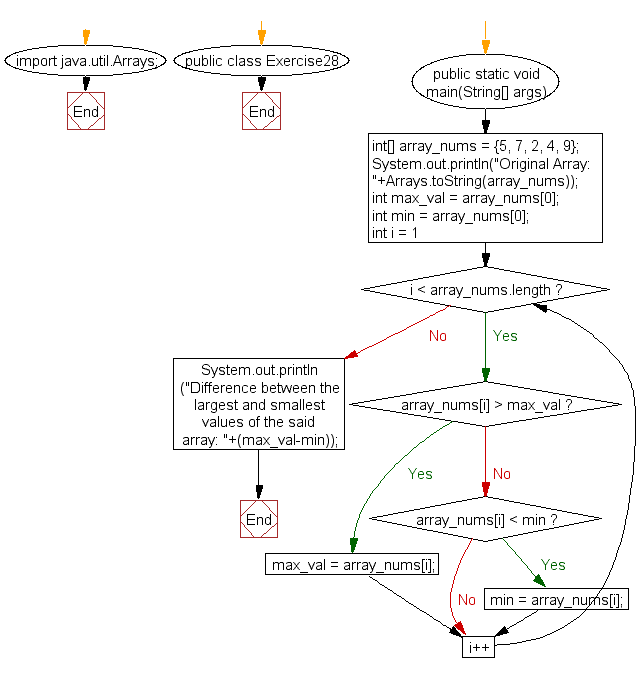
Java Code Editor:
Previous: Write a Java program to find the number of even and odd integers in a given array of integers.
Next: Write a Java program to compute the average value of an array of integers except the largest and smallest values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics