Java: Check if the number of 10 is greater than number to 20s in a given array of integers
Java Basic: Exercise-101 with Solution
Write a Java program to determine whether the number 10 in a given array of integers exceeds 20.
Pictorial Presentation:
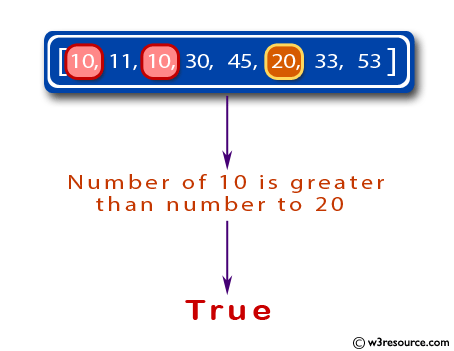
Sample Solution:
Java Code:
import java.util.*;
public class Exercise101 {
public static void main(String[] args) {
int[] array_nums = {10, 11, 10, 30, 45, 20, 33, 53};
int result = 0;
System.out.println("Original Array: "+Arrays.toString(array_nums));
int ctr1 = 0; // Initialize a counter to count occurrences of value 10
int ctr2 = 0; // Initialize a counter to count occurrences of value 20
for(int i = 0; i < array_nums.length; i++) {
if(array_nums[i] == 10)
ctr1++; // Increment ctr1 when the element is equal to 10
if(array_nums[i] == 20)
ctr2++; // Increment ctr2 when the element is equal to 20
}
System.out.printf(String.valueOf(ctr1 > ctr2)); // Check if the count of 10 is greater than the count of 20
System.out.printf("\n");
}
}
Sample Output:
Original Array: [10, 11, 10, 30, 45, 20, 33, 53] true
Flowchart:
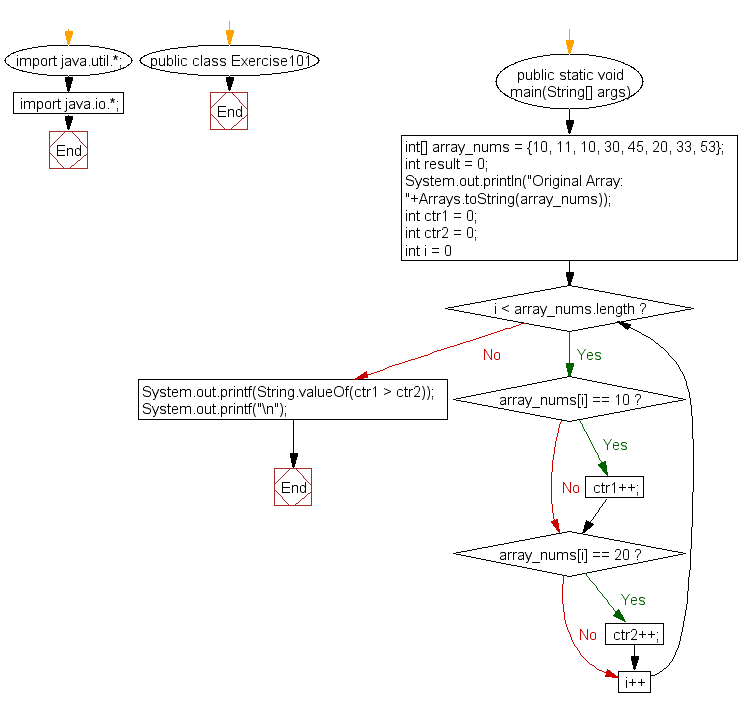
Java Code Editor:
Previous: Write a Java program to count the two elements of two given arrays of integers with same length, differ by 1 or less.
Next: Write a Java program to check if a specified array of integers contains 10 or 30.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics