Basic layout in JavaFX
JavaFx Basic: Exercise-3 with Solution
Write a JavaFX application with multiple components (buttons, labels, text fields) arranged in a VBox or HBox layout.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create labels, text fields, and buttons.
Label nameLabel = new Label("Name:");
TextField nameField = new TextField();
Label ageLabel = new Label("Age:");
TextField ageField = new TextField();
Button submitButton = new Button("Submit");
Button clearButton = new Button("Clear");
// Create a VBox layout to arrange the components vertically.
VBox root = new VBox(10); // 10 is the spacing between elements.
root.getChildren().addAll(nameLabel, nameField, ageLabel, ageField, submitButton, clearButton);
// Set action for the "Submit" button.
submitButton.setOnAction(event -> {
String name = nameField.getText();
String age = ageField.getText();
// You can perform an action with the input values, e.g., display them in an alert.
displayInfo("Information", "Name: " + name + "\nAge: " + age);
});
// Set action for the "Clear" button.
clearButton.setOnAction(event -> {
nameField.clear();
ageField.clear();
});
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200); // Width and height of the window.
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("VBox Layout App");
// Show the window.
primaryStage.show();
}
// Helper method to display information in an alert.
private void displayInfo(String title, String content) {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setContentText(content);
alert.showAndWait();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Create labels, text fields, and buttons for name, age, submit, and clear actions.
- Organize these components in a vertical layout (VBox) with 10 pixels of spacing between them.
- Set actions for the "Submit" and "Clear" buttons.
- The 'submitButton' action gets the text from the name and age text fields and displays this information in an alert dialog using the "displayInfo()" method.
The 'clearButton' action clears text fields.
- Create a 'Scene' with the layout, setting the window dimensions to 300x200.
- Set the window's title.
- Display the window.
- The "displayInfo()" method creates an information alert dialog to display a title and content message.
Sample Output:
Flowchart:
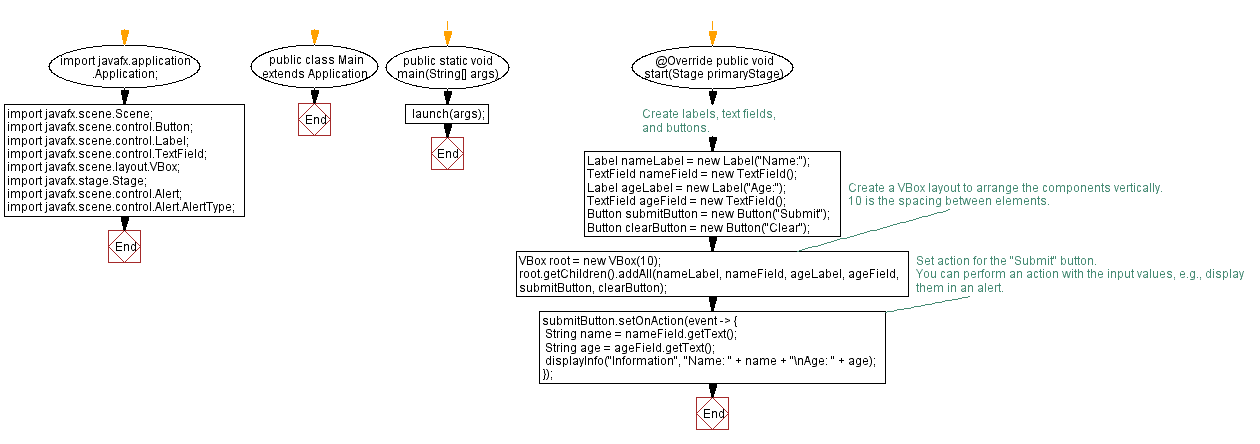
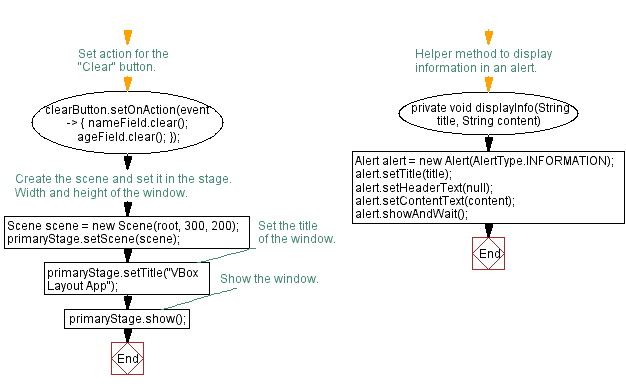
Java Code Editor:
Previous: Button click event in JavaFX.
Next: Text input and display in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics