Button click event in JavaFX
JavaFx Basic: Exercise-2 with Solution
Write a JavaFX application that creates a button. On clicking the button, display an alert.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a button.
Button actionButton = new Button("Click Me");
// Set an action when the button is clicked.
actionButton.setOnAction(event -> {
// You can choose to either display an alert:
showAlert("Button Clicked", "You clicked the button!");
// OR change the button text:
// actionButton.setText("Clicked!");
});
// Create a layout for the button.
VBox root = new VBox(10);
root.getChildren().add(actionButton);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 150);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Button Action App");
// Show the window.
primaryStage.show();
}
// Helper method to show an alert dialog.
private void showAlert(String title, String content) {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setContentText(content);
alert.showAndWait();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the start method:
- Create a button labeled "Click Me."
- Set an action to be executed when the button is clicked.
- The action attached to the button can do one of the following:
- Display an information alert with a title and content message.
- Change the text of the button (this part is commented out in the code).
- Create a layout (VBox) for the button with a spacing of 10 pixels.
- Create a Scene with the layout, setting the window dimensions to 300x150.
- Set the window's title to "Button Action App."
- Show the window.
Sample Output:
Flowchart:
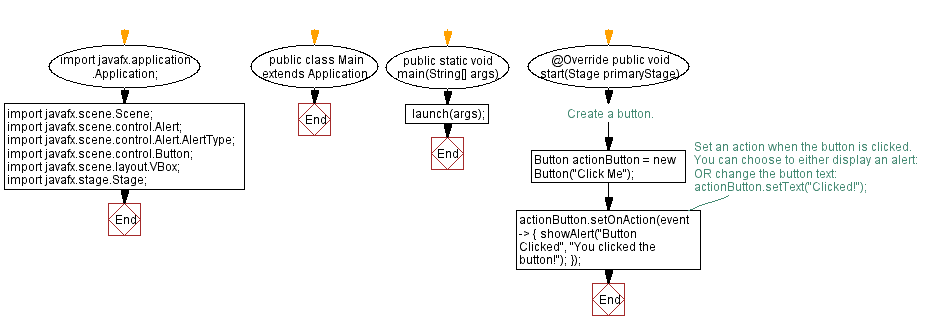
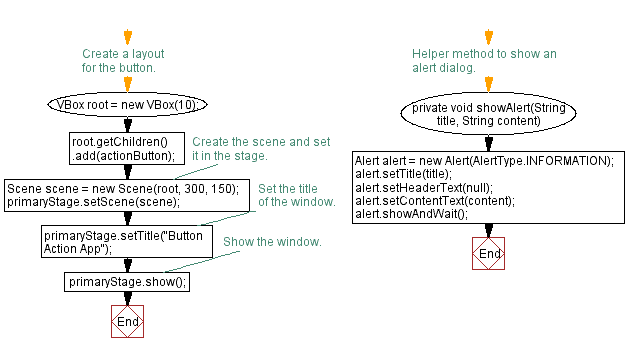
Java Code Editor:
Previous: Hello World JavaFX application.
Next: Basic layout in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics