Separating styling from logic in JavaFX with external CSS
JavaFx Styling and CSS: Exercise-5 with Solution
Write a JavaFX program that develops a JavaFX application and links an external CSS file to style the components. Explore how this separates styling from code logic.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Styled JavaFX App");
// Create buttons
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
// Add buttons to a VBox layout
VBox vbox = new VBox(10, button1, button2);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load external CSS file to style the components
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
/*styles.css*/
/* Styling for buttons */
.button {
-fx-font-size: 14px;
-fx-padding: 8px 12px;
-fx-background-color: #1E90FF;
-fx-text-fill: white;
-fx-border-color: #1E90FF;
-fx-border-radius: 5px;
}
.button:hover {
-fx-background-color: #32CD32; /* Change color on hover */
}
Explanation:
In the exercise above the JavaFX code creates a window containing two buttons styled using an external CSS file.
A brief explanation of the code follows:
- Setting up the UI: The start method initializes a JavaFX window (Stage) with the title "Styled JavaFX App". Two buttons (Button) labeled as "Button 1" and "Button 2" are created.
- Layout Structure: The buttons are added to a vertical box (VBox) layout with a spacing of 10 pixels between them using the VBox constructor.
- Applying CSS Styling: The buttons are styled using an external CSS file (styles.css). The CSS file specifies a class selector .button to define the default styling for the buttons. It includes properties such as font size, padding, background color, text color, border color, and border radius.
- Styling on Hover: Additionally, the CSS file defines a pseudo-class selector .button:hover, which changes the background color of the buttons to a different shade when the mouse hovers over them.
- Scene and Stylesheet: The scene (Scene) is created with the VBox layout and set to a size of 300x200 pixels. The external CSS file (styles.css) is loaded into the scene using scene.getStylesheets().add() to apply the specified styles to the buttons.
- Launching the Application: The main method launches the JavaFX application by calling launch(args).
Sample Output:
Flowchart:
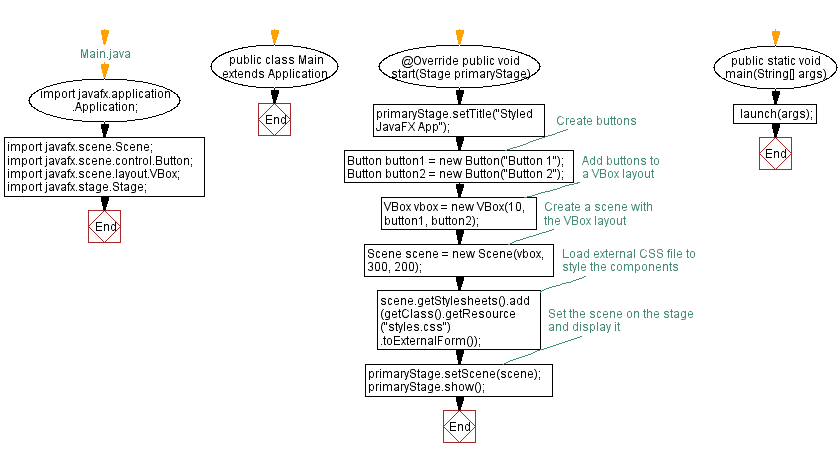

Java Code Editor:
Previous: Customizing JavaFX toggle buttons with CSS styling.
Next: JavaFX styling precedence: CSS vs inline styling override.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics