Styling JavaFX buttons with pseudo-classes
JavaFx Styling and CSS: Exercise-7 with Solution
Write a JavaFX program that uses pseudo-classes such as :hover or :focused in CSS to alter the appearance of buttons or text fields when the mouse hovers over them or they are in focus.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Pseudo-Class Example");
// Create buttons
Button button1 = new Button("Hover over me!");
Button button2 = new Button("Click me!");
// Set IDs for buttons (for CSS styling)
button1.setId("hover-button");
button2.setId("focused-button");
// Add buttons to VBox layout
VBox vbox = new VBox(button1, button2);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load external CSS file
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
/* styles.css */
/* Styling for the button when hovered */
#hover-button:hover {
-fx-background-color: #FF6347; /* Change background color on hover */
-fx-text-fill: white; /* Change text color on hover */
}
/* Styling for the button when focused */
#focused-button:focused {
-fx-background-color: #1E90FF; /* Change background color when focused */
-fx-text-fill: white; /* Change text color when focused */
}
Explanation:
In the above exercise, two buttons are created and placed in a vertical box layout. The first button (button1) changes its background color and text color when hovered over due to the :hover pseudo-class in the CSS file. The second button (button2) changes its background color and text color when it gains focus due to the :focused pseudo-class in the CSS file.
Sample Output:
Flowchart:
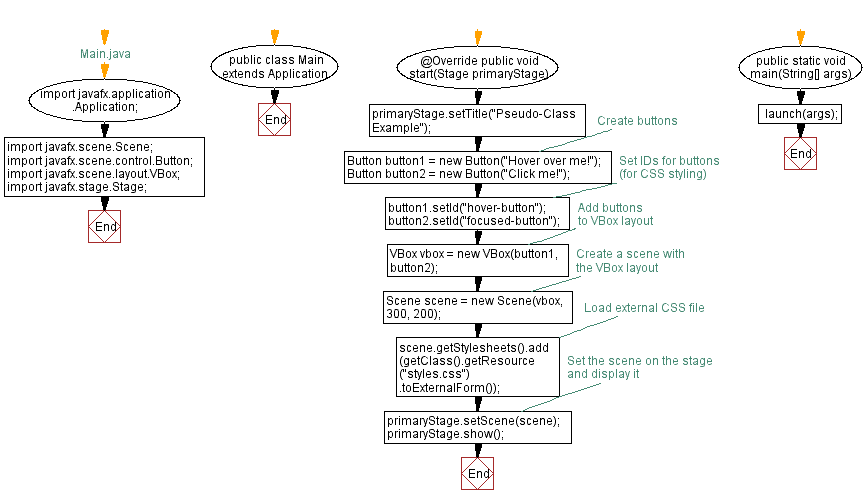
Java Code Editor:
Previous: JavaFX styling precedence: CSS vs inline styling override.
Next: Creating simple CSS animations in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics