Creating simple CSS animations in JavaFX
JavaFx Styling and CSS: Exercise-8 with Solution
Write a JavaFX program that implements simple animations or transitions using CSS on JavaFX components like buttons or rectangles. Modify properties like opacity, size, or position to create visual effects.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("CSS Animation Example");
// Create a button
Button button = new Button("Hover over me!");
// Set ID for the button (for CSS styling)
button.setId("animated-button");
// Add button to VBox layout
VBox vbox = new VBox(button);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load external CSS file
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
/* styles.css */
/* Styling for the button */
#animated-button {
-fx-background-color: #1E90FF; /* Initial background color */
-fx-text-fill: white; /* Text color */
-fx-padding: 10px 20px; /* Padding */
-fx-font-size: 14px; /* Font size */
-fx-transition: -fx-background-color 0.3s; /* Transition effect for background color */
}
/* Animation on hover */
#animated-button:hover {
-fx-background-color: #FF6347; /* Background color change on hover */
}
Explanation:
In the above exercise, a button is created and placed in a vertical box layout. The button's color changes smoothly when hovered over due to the CSS transition effect specified in the styles.css file.
The button's background color will smoothly transition when hovered over, creating an animation effect. You can modify the CSS properties and transition duration in the CSS file to experiment with different animation effects like opacity changes, size transformations, etc.
Sample Output:
Flowchart:
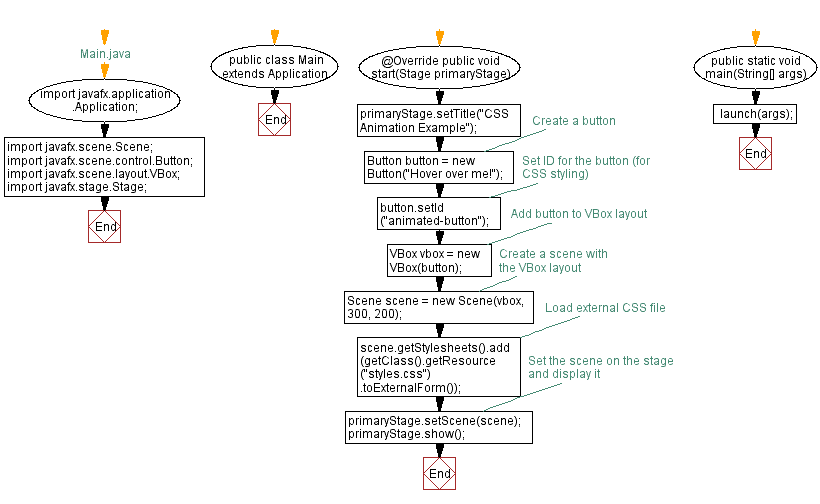
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/javafx/javafx-styling-and-css-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics