Java Multi Thread: Communication with Exchanger
Java Multithreading: Exercise-11 with Solution
Write a Java program that utilizes the Exchanger class for exchanging data between two threads.
Sample Solution:
Java Code:
import java.util.concurrent.Exchanger;
public class ExchangerExercise {
public static void main(String[] args) {
Exchanger < String > exchanger = new Exchanger < > ();
// Create and start two worker threads
Thread thread1 = new Thread(new Worker(exchanger, "Data from Thread 1"));
Thread thread2 = new Thread(new Worker(exchanger, "Data from Thread 2"));
thread1.start();
thread2.start();
}
static class Worker implements Runnable {
private final Exchanger < String > exchanger;
private final String data;
public Worker(Exchanger < String > exchanger, String data) {
this.exchanger = exchanger;
this.data = data;
}
@Override
public void run() {
try {
System.out.println(Thread.currentThread().getName() + " sending data: " + data);
String receivedData = exchanger.exchange(data); // Exchange data with the other thread
System.out.println(Thread.currentThread().getName() + " received data: " + receivedData);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
Sample Output:
Thread-1 sending data: Data from Thread 2 Thread-0 sending data: Data from Thread 1 Thread-0 received data: Data from Thread 2 Thread-1 received data: Data from Thread 1
Explanation:
In the above exercise, we create an Exchanger<String> instance for exchanging data between two threads. Each worker thread sends its own data and receives it from the other thread using the exchange() method of the Exchanger. The threads will block until both threads arrive at the exchange point.
When we run this program, you'll see that each thread sends its own data and receives the data sent by the other thread through the Exchanger. This demonstrates the exchange of data between two threads.
Flowchart:
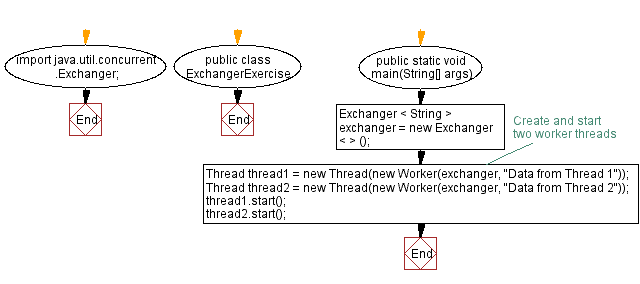
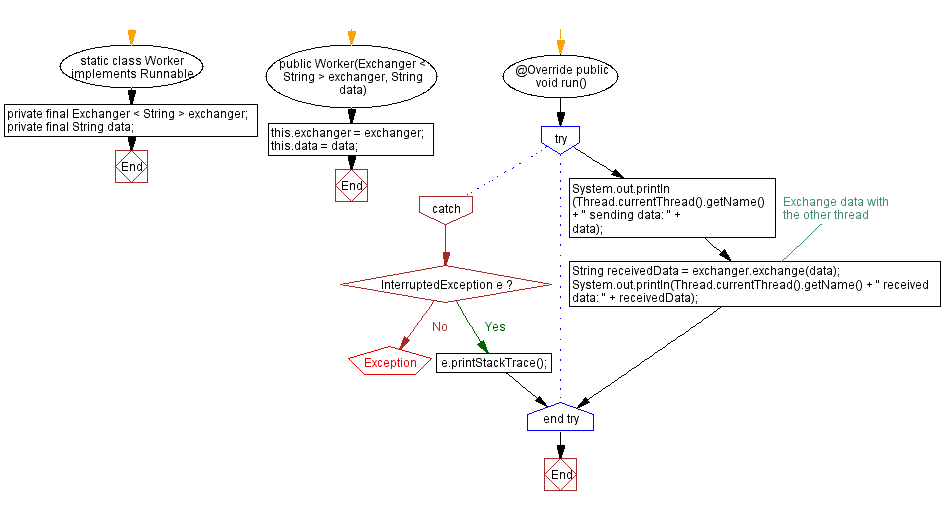
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Synchronize multiple threads.
Next: Asynchronous Task Execution in Java with Callable and Future.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics