Asynchronous Task Execution in Java with Callable and Future
Java Multithreading: Exercise-12 with Solution
Write a Java program to demonstrate the usage of the Callable and Future interfaces for executing tasks asynchronously and obtaining their results.
Sample Solution:
Java Code:
import java.util.concurrent.*;
public class CallableFutureExercise {
public static void main(String[] args) {
// Create a thread pool with a single worker thread
ExecutorService executor = Executors.newSingleThreadExecutor();
// Submit a Callable task to the executor
Future < String > future = executor.submit(new Task());
// Perform other operations while the task is executing
try {
// Wait for the task to complete and get the result
String result = future.get();
System.out.println("Task result: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
// Shutdown the executor
executor.shutdown();
}
static class Task implements Callable < String > {
@Override
public String call() throws Exception {
// Perform the task and return the result
Thread.sleep(2000); // Simulate some time-consuming operation
return "Task completed!";
}
}
}
Sample Output:
Task result: Task completed!
Explanation:
In the above exercise -
- First we create a Callable task called "Task" that performs a time-consuming operation and returns a String result. We then create an ExecutorService with a single worker thread using the Executors.newSingleThreadExecutor() method.
- We submit the Task to the executor using the submit() method, which returns a Future object representing the task result. We can continue performing other operations while the task executes.
- To obtain the result of the task, we call the get() method on the Future object. This method blocks until the task is complete and returns the result. We handle possible exceptions like InterruptedException and ExecutionException that may occur during the execution or retrieval of the result.
- Finally, we shutdown the executor using the shutdown() method to release its resources.
Flowchart:
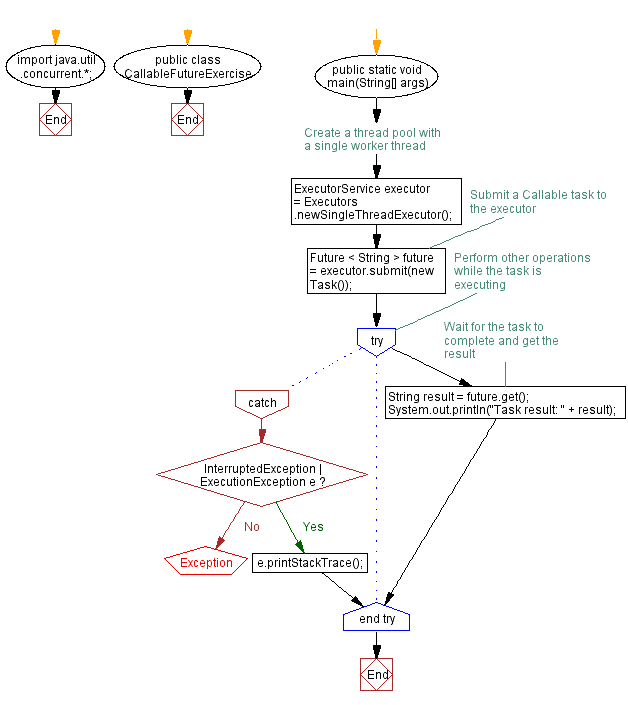
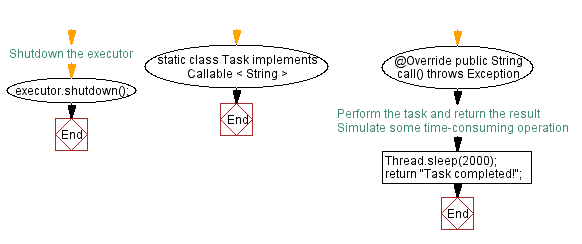
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Communication with Exchanger.
Next: Java Scheduled Task Execution with ScheduledExecutorService.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/multithreading/java-multithreading-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics