Java: Append two given strings such that, if the concatenation creates a double characters then omit one of the characters
Java String: Exercise-56 with Solution
Write a Java program that appends two strings, omitting one character if the concatenation creates double characters.
Visual Presentation:
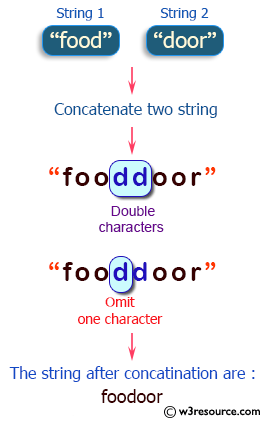
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to concatenate two strings based on specific conditions
public String conCat(String st1, String st2) {
// Check if both strings are not empty and the last character of the first string matches the first character of the second string
if (st1.length() != 0 && st2.length() != 0 && st1.charAt(st1.length() - 1) == st2.charAt(0))
return st1 + st2.substring(1); // Concatenate the strings excluding the duplicate character
return st1 + st2; // If no match or empty strings, concatenate the strings as they are
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
// Define two strings for concatenation
String str1 = "food";
String str2 = "door";
// Display the given strings and the concatenated string using the conCat method
System.out.println("The given strings are: " + str1 + " and " + str2);
System.out.println("The string after concatenation is: " + m.conCat(str1, str2));
}
}
Sample Output:
The given strings are: food and door The string after concatination are: foodoor
Flowchart:
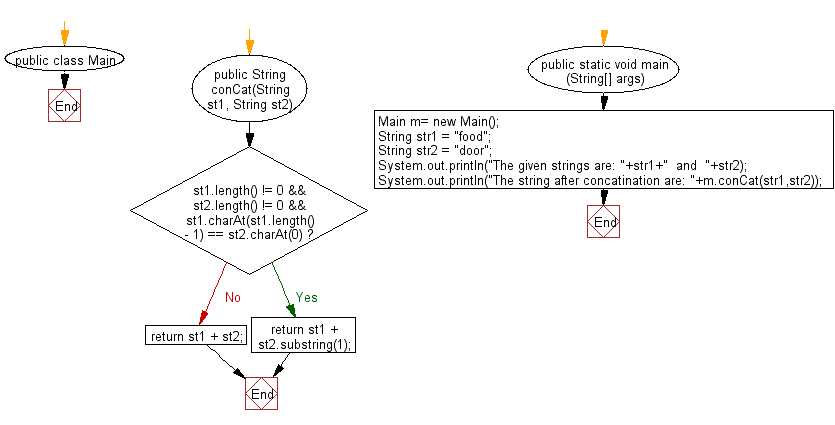
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to remove all adjacent duplicates recursively from a given string.
Next: Write a Java program to create a new string from a given string swapping the last two characters of the given string. The length of the given string must be two or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics