Java: Create a new string from a given string swapping the last two characters of the given string
Java String: Exercise-57 with Solution
Write a Java program to create a string from a given string by swapping the last two characters of the given string. The string length must be two or more.
Visual Presentation:
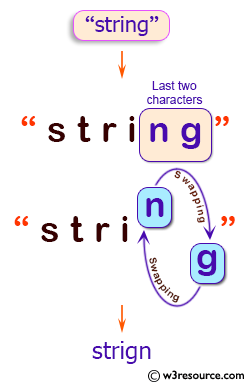
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to swap the last two characters of a string
public String lastTwo(String str) {
// Check if the string has less than two characters, return the string as is
if (str.length() < 2) return str;
// Swap the last two characters of the string and return the modified string
return str.substring(0, str.length() - 2) + str.charAt(str.length() - 1) + str.charAt(str.length() - 2);
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
// Define a string for swapping the last two characters
String str1 = "string";
// Display the given string and the string after swapping its last two characters using the lastTwo method
System.out.println("The given string is: " + str1);
System.out.println("The string after swapping the last two characters is: " + m.lastTwo(str1));
}
}
Sample Output:
The given strings is: string The string after swap last two characters are: strign
Flowchart:
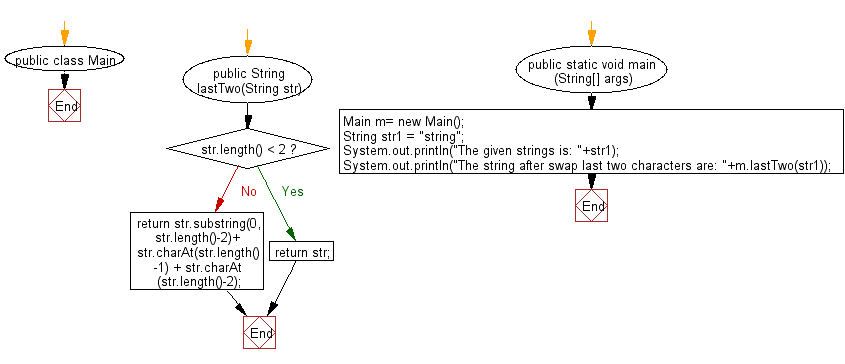
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to append two given strings such that, if the concatenation creates a double characters then omit one of the characters.
Next: Write a Java program to read a string and return true if it ends with a specified string of length 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/string/java-string-exercise-57.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics