JavaScript: Create a case-insensitive search
JavaScript String: Exercise-38 with Solution
Write a JavaScript function to create a case-insensitive search.
Test Data:
console.log(case_insensitive_search('JavaScript Exercises', 'exercises'));
"Matched"
console.log(case_insensitive_search('JavaScript Exercises', 'Exercises'));
"Matched"
console.log(case_insensitive_search('JavaScript Exercises', 'Exercisess'));
"Not Matched"
Visual Presentation:
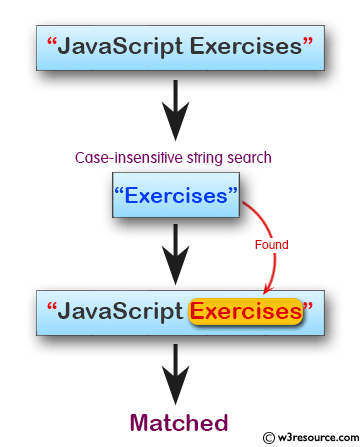
Sample Solution:
JavaScript Code:
// Define a function called case_insensitive_search which takes two parameters: str (the string to search within) and search_str (the string to search for)
function case_insensitive_search(str, search_str)
{
// Use a regular expression with the "i" flag to perform a case-insensitive search for search_str within str, and store the result in the variable result
var result= str.search(new RegExp(search_str, "i"));
// If the result is greater than 0 (indicating a match), return 'Matched'; otherwise, return 'Not Matched'
if (result>0)
return 'Matched';
else
return 'Not Matched';
}
// Call the case_insensitive_search function with different strings and search terms, and print the results to the console
console.log(case_insensitive_search('JavaScript Exercises', 'exercises'));
console.log(case_insensitive_search('JavaScript Exercises', 'Exercises'));
console.log(case_insensitive_search('JavaScript Exercises', 'Exercisess'));
Output:
Matched Matched Not Matched
Explanation:
The above code defines a function called "case_insensitive_search()" that performs a case-insensitive search for a given 'search_str' within a larger 'str'.
It uses the "search()" method with a regular expression constructed using 'RegExp' with the "i" flag to indicate case insensitivity.
If a match is found, it returns 'Matched', otherwise 'Not Matched'. The function is then called with different input strings and search terms, and the results are printed to the console.
Flowchart:
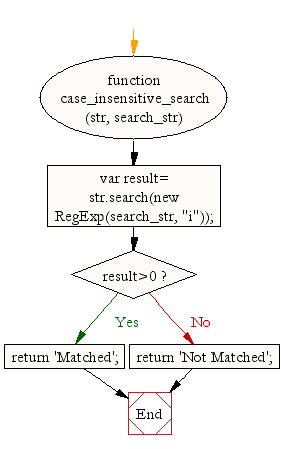
Live Demo:
See the Pen JavaScript Create a case-insensitive search-string-ex-38 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to test case insensitive (except special Unicode characters) string comparison.
Next: Write a JavaScript function to uncapitalize the first character of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics