Node.js REPL (Read-Eval-Print Loop)
Introduction
A Read-Eval-Print Loop (REPL) is a simple, interactive computer programming environment. The term 'REPL' is usually used to refer to a LISP interactive environment but can be applied to command line shells and similar environments for programming languages like Python, Ruby etc. In this tutorial, we have discussed REPL with respect to Node.js. In a REPL environment, an user can enter one and more expressions, which are then evaluated (bypassing the compile stage), and the result displayed. There are 4 components to a REPL (comes from the names of the Lisp primitive functions ) :
- A read function, which accepts an expression from the user and parses it into a data structure in memory .
- An eval function, which takes the data structure and evaluates.
- A print function, which prints the result.
- A loop function, which runs the above three commands until termination
Node.js ships with a REPL. If you start the Node.js binary without any arguments, you will see the REPL command prompt, the > character. The node.js REPL works exactly the same as Chrome’s REPL, so from this prompt, you can type any Javascript command you wish. It is extremely useful for experimenting with node.js and debugging JavaScript code.
REPL Features
- Press Ctrl+C to terminate the current command.
- Pressing Ctrl + C twice causes the REPL to quit.
- Press Ctrl+D to exit the REPL.
- Pressing up and down arrow keys you can see command history and modify previous commands.
- Press tab key to see the list of current commands. If you type a single character followed by tab it will show the list of keywords, functions, and variables starting with that particular character.
- The REPL can handle multiline expression.
- The REPL provides a special variable _ (underscore) which is used to pull the result of the last expression.
Whenever you type a command, it will print the return value of the command. See the following examples :
Simple calculation :
> 1+2
3
> 1+2-3
0
> 1+(3-2)*4/2
3
>
In the following codes, two variables x and y have declared with initial values 10 and 20. In both cases, REPL prints "undefined" because the variable declaration statements do not return any value. The next statement x+y returns the value 30. After execution of console.log(x+y) function, REPL prints the value of x+y and displays "undefined" again because console.log() function returns no value.
> var x = 10;
undefined
> var y = 20;
undefined
> x+y
30
>console.log(x+y);
30
undefined
>
Example: List of current commands:
Press tab key to see the list of current commands.
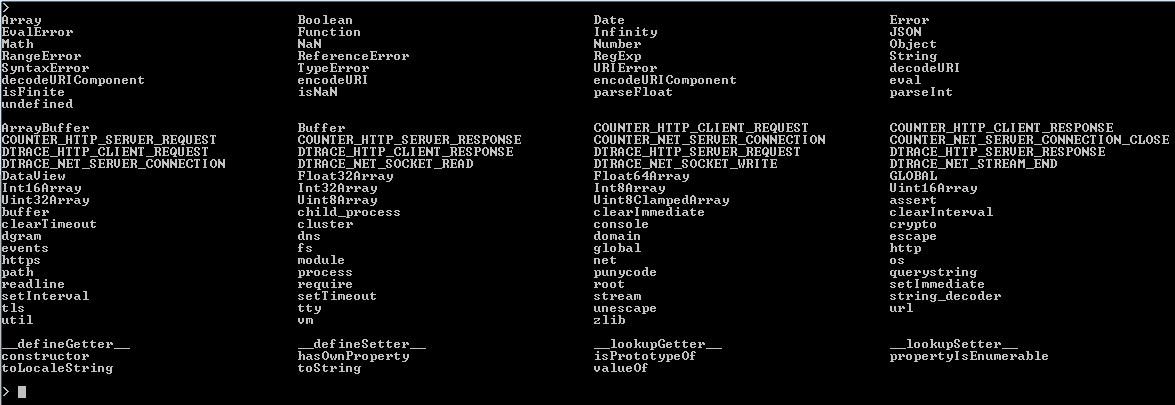
In the following list character d is pressed followed by the tab and the REPL displays the list of keywords, functions, and variables starting with that particular character.
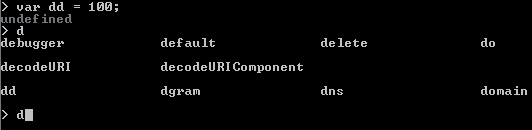
Example: Multiline expression
The REPL can handle multiline expression smoothly. Start to enter a command, function or another unclosed tag into the REPL, and the REPL automatically extends to multiple lines. See the following example :
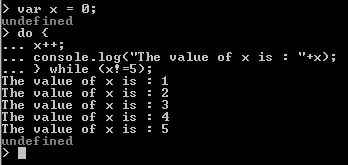
Note : The ... is used by the REPL to indicate a multiline expression.
Example: special variable _ (underscore)
The special variable _ (underscore) contains the result of the last expression. In the following example, _ variable first display the length of the declared array i.e. 6 and again display 8 after adding 2 to it.
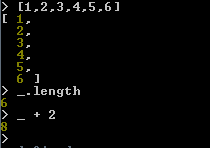
REPL Commands:
.help:
The .help command is used to display all the available REPL commands.

.break:
The .break command is used to exit from a multi-line expression, the command is useful if you make something wrong or do not want to complete or want to start over again. In the following example, a .break command is used to terminate a do..while loop before completing.
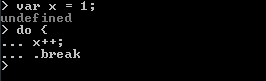
.clear:
The .clear command is used to exit from a multi-line expression, similar to .break.
.save:
The .save command is used to save the current REPL session to a file. In the following example, the current session is saved to the file test.js.
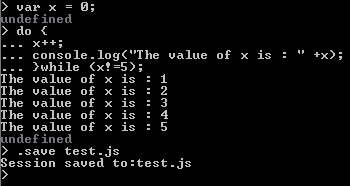
Content of test.js
var x = 0;
do {
x++;
console.log("The value of x is : " +x);
}while (x!=5);
.load:
The .load command is used to load the contents of a file into the current REPL session. In the following example .load command loads previously saved file test.js.
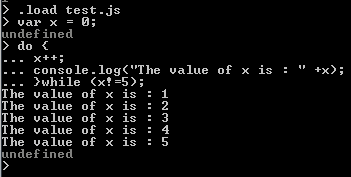
Previous:
Programming Model
Next:
Node Package
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics