Set PHP session timeout: 30 minutes of inactivity
PHP Cookies and Sessions: Exercise-11 with Solution
Write a PHP script to set a session timeout after 30 minutes of inactivity.
Sample Solution:
PHP Code :
<?php
session_save_path('i:/custom/');
session_start();
// Set the session timeout duration in seconds
$sessionTimeout = 1800; // 30 minutes
// Check if the session has already been started and calculate the time since the last activity
if (isset($_SESSION['LAST_ACTIVITY'])) {
$lastActivity = $_SESSION['LAST_ACTIVITY'];
$currentTime = time();
$timeSinceLastActivity = $currentTime - $lastActivity;
// Check if the session has exceeded the timeout duration
if ($timeSinceLastActivity > $sessionTimeout) {
// Session expired, destroy the session
session_unset();
session_destroy();
echo "Session expired. Please log in again.";
} else {
// Update the last activity time
$_SESSION['LAST_ACTIVITY'] = $currentTime;
echo "Session active.";
}
} else {
// Set the last activity time for the session
$_SESSION['LAST_ACTIVITY'] = time();
echo "Session started.";
}
?>
Sample Output:
Session started.
After few seconds Session active.
Explanation:
In the above exercise -
- Start the session using session_start() to initialize the session.
- Define the session timeout duration in seconds. In this case, it's set to 1800 seconds (30 minutes).
- Check if the session has already been started by verifying if the LAST_ACTIVITY session variable is set. If it is set, it means the session is active, and we check the time since the last activity.
- Inside the if block, we calculate the time since the last activity by subtracting the stored LAST_ACTIVITY value from the current time using time(). This gives us the variable $timeSinceLastActivity.
- We then compare $timeSinceLastActivity with the session timeout duration. If it exceeds the timeout, we destroy the session using session_unset() and session_destroy(), and display the message "Session expired. Please log in again."
- If the session is still active (within the timeout duration), we update the LAST_ACTIVITY session variable to the current time and display the message "Session active."
- If the session has not been started yet, we set the LAST_ACTIVITY session variable to the current time using time() and display the message "Session started."
Flowchart:
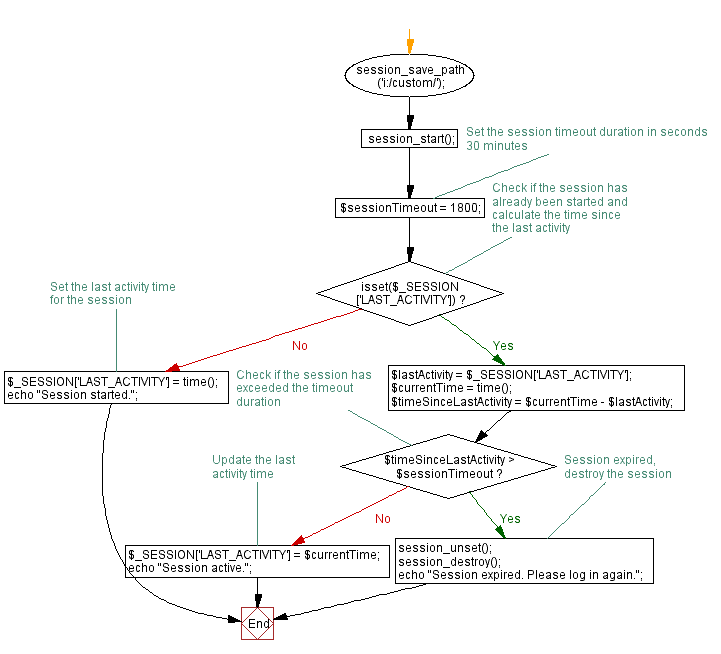
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Retrieve and display user preferences in PHP session variable.
Next: Display active session count on server.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics