PHP Function: Handling empty string exception
PHP Exception Handling: Exercise-6 with Solution
Write a PHP function that takes a string as input and throws an exception if the string is empty.
Sample Solution:
PHP Code :
<?php
function validateString($inputString) {
if (empty($inputString)) {
throw new Exception("String should not be empty!");
}
}
try {
$string = "";
validateString($string);
echo "Valid string: " . $string;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: String should not be empty
Explanation:
In the above exercise, we have a function validateString() that takes a string $inputString as input. Inside the function, we use the empty() function to check if the string is empty. If it is, we throw a new instance of the Exception class with the message "String should not be empty!"
In the main code, we call the validateString() function with an empty string. Since the string is empty, it throws an exception. The exception is caught in the catch block, and the error message is displayed using $e->getMessage ().
Flowchart:
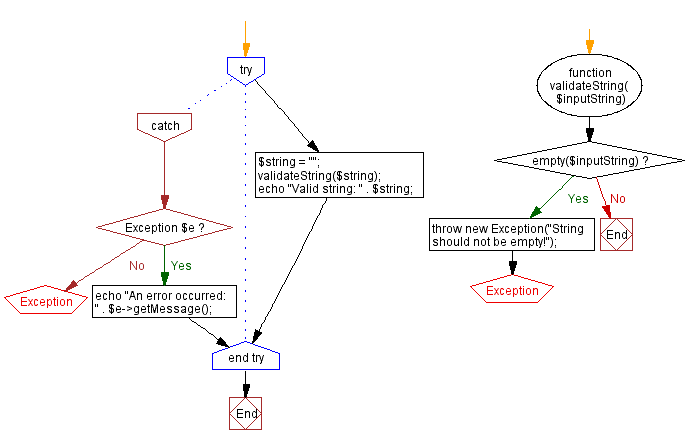
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Custom exception for missing file.
Next: Multiple catch blocks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics