PHP Exception Handling: Multiple catch blocks
PHP Exception Handling: Exercise-7 with Solution
Write a PHP program that implements multiple catch blocks to handle different types of exceptions.
Sample Solution:
PHP Code :
<?php
try {
// Code that may throw different types of exceptions
$number = "abc";
if (!is_numeric($number)) {
throw new InvalidArgumentException("Invalid number.");
}
$result = 100 / $number;
echo "Result: " . $result;
} catch (InvalidArgumentException $e) {
// Handle InvalidArgumentException
echo "InvalidArgumentException: " . $e->getMessage();
} catch (DivisionByZeroError $e) {
// Handle DivisionByZeroError
echo "DivisionByZeroError: " . $e->getMessage();
} catch (Throwable $e) {
// Handle generic Throwable (catches any other type of exception)
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
InvalidArgumentException: Invalid number.
Explanation:
In the above exercise,
We have a try block that contains code that throws different types of exceptions. We first check if the $number is numeric using is_numeric() function and throw an InvalidArgumentException if it's not.
Then, we attempt a division operation with $number to trigger a DivisionByZeroError if the number is zero.
The catch blocks are defined to handle specific exception types.
- The first catch block catches InvalidArgumentException and displays an appropriate error message.
- The second catch block catches DivisionByZeroError and handles it accordingly.
- The third catch block catches Throwable, which is the base class for all exceptions, and handles any other type of exception that may occur.
Flowchart:
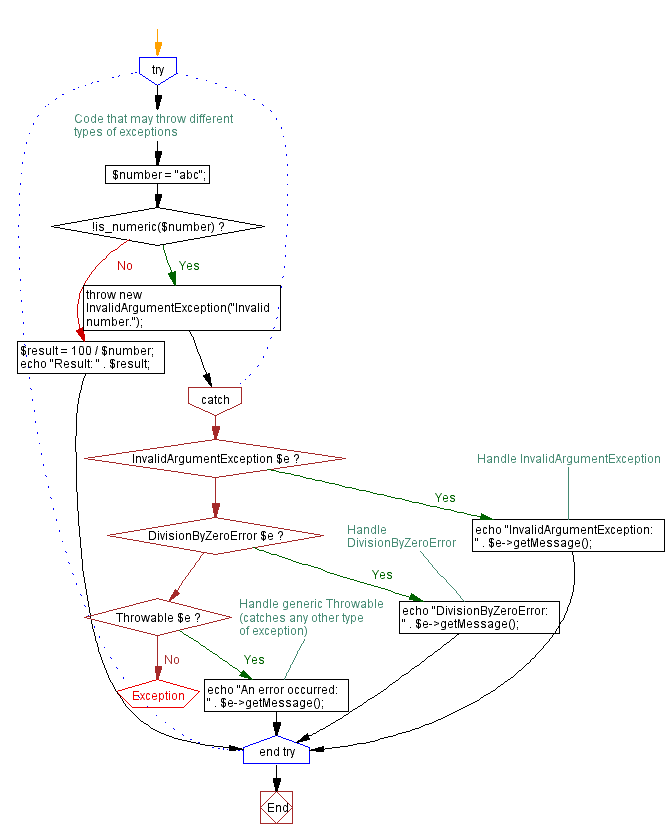
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Handling empty string exception.
Next: Exception handling.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics