PHP exception handling with the finally block
PHP Exception Handling: Exercise-9 with Solution
Write a PHP script that implements the use of the finally block in exception handling.
Sample Solution:
PHP Code :
<?php
try {
// Code that may throw an exception
$number = 0;
if ($number === 0) {
throw new Exception("Number cannot be zero.");
}
$result = 200 / $number;
echo "Result: " . $result;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
} finally {
// Code that will always be executed
echo "This will always be executed.";
}
?>
Sample Output:
An error occurred: Number cannot be zero.This will always be executed.
Explanation:
In the above exercise,
- We have a try block that contains code that may throw an exception. We check if the $number is zero and throw an exception if it is.
- The catch block catches the Exception and displays an appropriate error message using $e->getMessage().
- The finally block is where you can include code that will always be executed, regardless of whether an exception was thrown or not. This example simply echos the message "This will always be executed."
Flowchart:
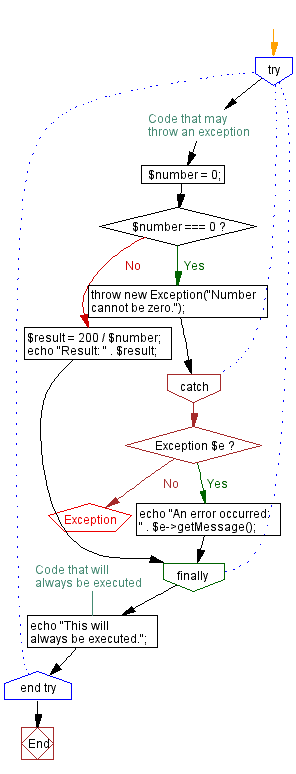
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Exception handling.
Next: Handling specific exceptions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics