PHP function to write string to file
PHP File Handling: Exercise-4 with Solution
Write a PHP function to write a string to a file.
Sample Solution:
PHP Code :
<?php
function writeToFile($filename, $content) {
try {
$fileHandle = fopen($filename, 'w');
if ($fileHandle === false) {
throw new Exception("Error opening the file for writing.");
}
fwrite($fileHandle, $content);
fclose($fileHandle);
echo "Content written to the file successfully.";
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
}
// Usage:
$filename = "i:/example.txt";
$content = "PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.";
writeToFile($filename, $content);
?>
Sample Output:
Content written to the file successfully.
Explanation:
In the above exercise -
- The writeToFile() function takes two parameters: $filename (the name of the file to write to) and $content (the string to be written).
- Inside the function, we use fopen() with the 'w' mode to open the file for writing. If there is an error opening the file, we throw an exception with the error message "Error opening the file for writing."
- We then use fwrite() to write the $content string to the file.
- After writing the content, we close the file using fclose().
- The content of the file is written if everything goes well.
Flowchart:
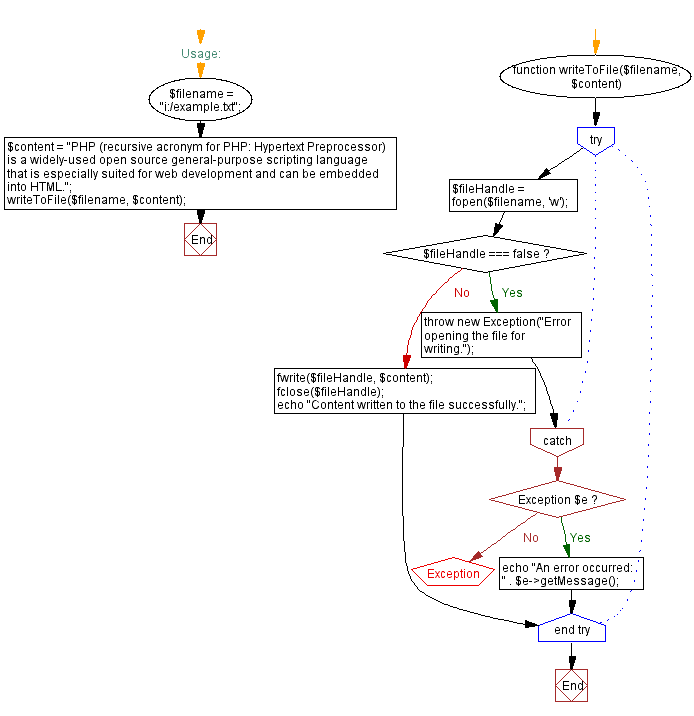
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP script to count lines in a text file.
Next: PHP program to read and display CSV data.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics