PHP OOP: Class with magic method
PHP OOP: Exercise-10 with Solution
Write a PHP class called 'Person' with properties like 'name' and 'age'. Implement the '__toString()' magic method to display person information.
Sample Solution:
PHP Code :
<?php
class Person {
private $name;
private $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function __toString() {
return "Name: " . $this->name . "</br>" .
"Age: " . $this->age . "</br>";
}
}
$person = new Person("Natalius Esther", 30);
echo $person;
?>
Sample Output:
Name: Natalius Esther Age: 30
Explanation:
In the above exercise -
- The Person class has two private properties: $name and $age, which represent the person's name and age.
- The constructor method __construct() is used to initialize the values of the properties when creating a new instance of the Person class.
- The __toString() magic method returns a string representation of the person's information when the object is treated as a string. It combines name and age properties into a formatted string.
Flowchart:
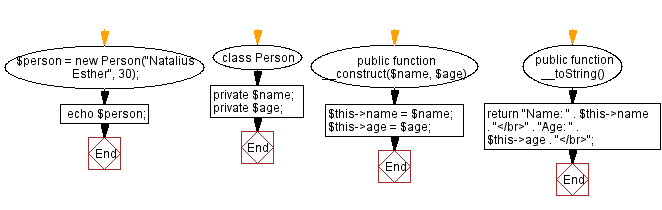
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract class for animal.
Next: PHP class inheritance with extended class.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics