PHP class for mathematical calculations
PHP OOP: Exercise-14 with Solution
Write a class called 'Math' with static methods like 'add()', 'subtract()', and 'multiply()'. Use these methods to perform mathematical calculations.
Sample Solution:
PHP Code :
<?php
class Math {
public static function add($num1, $num2) {
return $num1 + $num2;
}
public static function subtract($num1, $num2) {
return $num1 - $num2;
}
public static function multiply($num1, $num2) {
return $num1 * $num2;
}
}
$result1 = Math::add(4, 3);
$result2 = Math::subtract(14, 4);
$result3 = Math::multiply(8, 2);
echo "Addition: " . $result1 . "</br>";
echo "Subtraction: " . $result2 . "</br>";
echo "Multiplication: " . $result3 . "</br>";
?>
Sample Output:
Addition: 7 Subtraction: 10 Multiplication: 16
Explanation:
In the above exercise -
- The "Math" class has three static methods: add(), subtract(), and multiply(), which perform addition, subtraction, and multiplication respectively.
- Each method takes two parameters $num1 and $num2, performs the corresponding mathematical operation, and returns the result.
Flowchart:
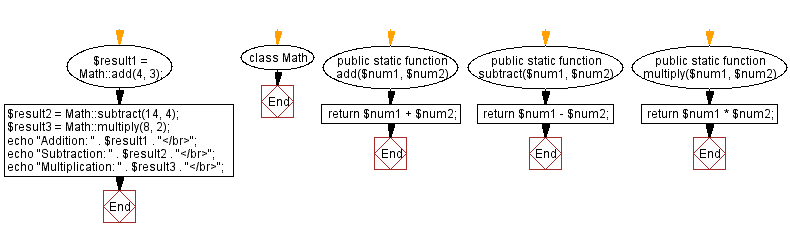
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class with a static property.
Next: PHP class for file operations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics