PHP class with a static property
PHP OOP: Exercise-13 with Solution
Write a class called 'Logger' with a static property called 'logCount' that keeps track of the number of log messages. Implement a static method to log a message.
Sample Solution:
PHP Code :
<?php
class Logger {
public static $logCount = 0;
public static function log($message) {
echo $message . "</br>";
self::$logCount++;
}
}
Logger::log("Log message 1");
Logger::log("Log message 2");
Logger::log("Log message 3");
echo "Total log count: " . Logger::$logCount . "</br>";
?>
Sample Output:
Log message 1 Log message 2 Log message 3 Total log count: 3
Explanation:
In the above exercise -
- The "Logger" class has a static property called $logCount, which is initially set to 0 and will be incremented each time a log message is logged.
- The log() method is a static method that takes a $message parameter and echoes the message. It also increments the logCount property using self::$logCount to refer to the static property.
Flowchart:
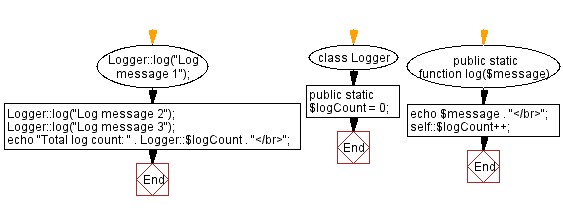
s
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class with comparable interface.
Next: PHP class for mathematical calculations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/oop/php-oop-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics