PHP class vehicle: Display vehicle details
PHP OOP: Exercise-5 with Solution
Write a PHP class called 'Vehicle' with properties like 'brand', 'model', and 'year'. Implement a method to display the vehicle details.
Sample Solution:
PHP Code :
<?php
class Vehicle {
private $brand;
private $model;
private $year;
public function __construct($brand, $model, $year) {
$this->brand = $brand;
$this->model = $model;
$this->year = $year;
}
public function displayDetails() {
echo "Brand: " . $this->brand . "</br>";
echo "Model: " . $this->model . "</br>";
echo "Year: " . $this->year . "</br>";
}
}
$car = new Vehicle("Ford", "F-150", 2020);
$car->displayDetails();
?>
Sample Output:
Brand: Ford Model: F-150 Year: 2020
Explanation:
In the above exercise -
- The "Vehicle" class has three private properties: $brand, $model, and $year, which represent the brand, model, and manufacturing year of the vehicle.
- The constructor method "__construct()" is used to initialize the values of the properties when creating a new instance of the Vehicle class.
- The "displayDetails()" method displays vehicle details by echoing the properties values.
Flowchart:
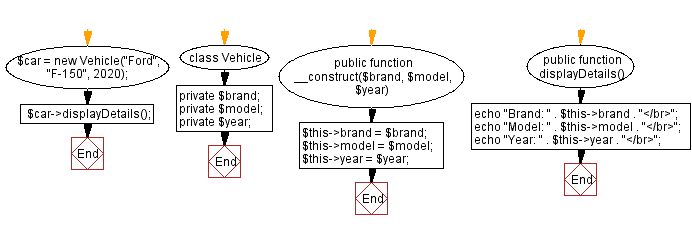
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Resizing functionality in the square class.
Next: PHP class hierarchy for library system.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics