PHP class for bank accounts: Deposit and withdraw
PHP OOP: Exercise-8 with Solution
Write a PHP a class called "BankAccount" with properties like "accountNumber" and "balance". Implement methods to deposit and withdraw money from the account.
Sample Solution:
PHP Code :
<?php
class BankAccount {
private $accountNumber;
private $balance;
public function __construct($accountNumber) {
$this->accountNumber = $accountNumber;
$this->balance = 0;
}
public function getAccountNumber() {
return $this->accountNumber;
}
public function getBalance() {
return $this->balance;
}
public function deposit($amount) {
$this->balance += $amount;
echo "Deposited $amount into account $this->accountNumber. New balance: $this->balance</br>";
}
public function withdraw($amount) {
if ($this->balance >= $amount) {
$this->balance -= $amount;
echo "Withdrawn $amount from account $this->accountNumber. New balance: $this->balance</br>";
}
else
{
echo "Want to withdrawn $amount?</br>";
echo "Insufficient balance in account $this->accountNumber. Current balance: $this->balance</br>";
}
}
}
$account = new BankAccount("SB-1234");
echo "Account Number: " . $account->getAccountNumber() . "</br>";
echo "Initial Balance: " . $account->getBalance() . "</br>";
$account->deposit(1000);
$account->withdraw(600);
$account->withdraw(700);
?>
Sample Output:
Account Number: SB-1234 Initial Balance: 0 Deposited 1000 into account SB-1234. New balance: 1000 Withdrawn 600 from account SB-1234. New balance: 400 Want to withdrawn 700? Insufficient balance in account SB-1234. Current balance: 400
Explanation:
In the above exercise -
- The "BankAccount" class has two private properties: $accountNumber and $balance, which represent the account number and current balance of the bank account, respectively.
- The constructor method __construct() initializes the accountNumber property and set the initial balance to 0.
- The "getAccountNumber()" and getBalance() methods are implemented to retrieve the account number and balance, respectively.
- The deposit($amount) method is used to deposit money into the account. It increases the balance by the specified amount and displays the new balance.
- The withdraw($amount) method withdraws money from the account. If the account has a sufficient balance, it decreases the balance by the specified amount and displays the new balance. Otherwise, it shows a message indicating an insufficient balance.
Flowchart:

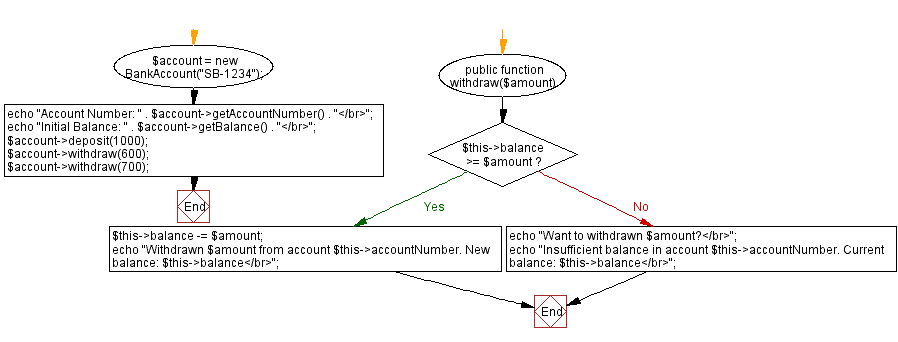
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class for student information.
Next: Abstract class for animal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics