PHP OOP: Abstract class for animal
PHP OOP: Exercise-9 with Solution
Write a PHP abstract class called 'Animal' with abstract methods like 'eat()' and 'makeSound()'. Create subclasses like 'Dog', 'Cat', and 'Bird' that implement these methods.
Sample Solution:
PHP Code :
<?php
abstract class Animal
{
abstract public function eat();
abstract public function makeSound();
}
class Dog extends Animal
{
public function eat()
{
echo "Dog is eating.</br>";
}
public function makeSound()
{
echo "Dog is barking.</br>";
}
}
class Cat extends Animal
{
public function eat()
{
echo "Cat is eating.</br>";
}
public function makeSound() {
echo "Cat is meowing.</br>";
}
}
class Bird extends Animal
{
public function eat()
{
echo "Bird is eating.</br>";
}
public function makeSound()
{
echo "Bird is chirping.</br>";
}
}
$dog = new Dog();
$dog->eat();
$dog->makeSound();
$cat = new Cat();
$cat->eat();
$cat->makeSound();
$bird = new Bird();
$bird->eat();
$bird->makeSound();
?>
Sample Output:
Dog is eating. Dog is barking. Cat is eating. Cat is meowing. Bird is eating. Bird is chirping.
Explanation:
In the above exercise -
- The "Animal" class is declared an abstract class, meaning it cannot be instantiated directly. It contains abstract methods eat() and makeSound() which are declared but not implemented.
- The "Dog", "Cat", and "Bird" classes are subclasses of "Animal" and implement the abstract methods eat() and makeSound() as required by the parent abstract class.
Flowchart:
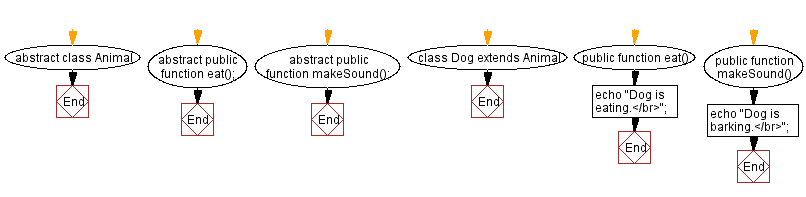
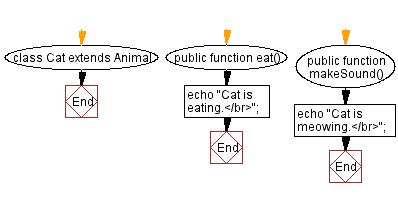
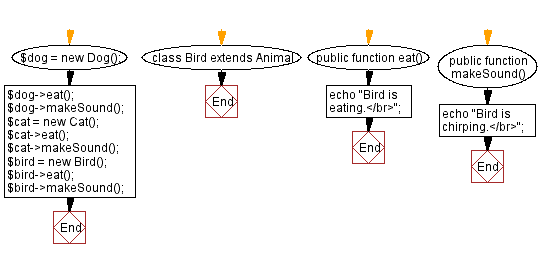
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class for bank accounts: Deposit and withdraw.
Next: Class with magic method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics