PHP function Exercises: Reverse a string
PHP function: Exercise-3 with Solution
Write a PHP function to reverse a string.
Visual Presentation:
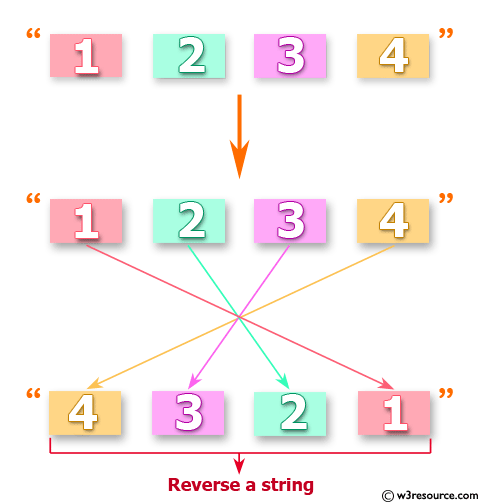
Sample Salution:
PHP Code:
<?php
// Function to reverse a string recursively
function reverse_string($str1)
{
// Get the length of the string
$n = strlen($str1);
// Base case: if the string has only one character, return the string itself
if ($n == 1)
{
return $str1;
}
else
{
// Decrement the length of the string
$n--;
// Recursively reverse the substring starting from the second character
// and concatenate the first character to it
return reverse_string(substr($str1, 1, $n)) . substr($str1, 0, 1);
}
}
// Call the reverse_string function with the argument '1234' and print the result
print_r(reverse_string('1234') . "\n");
?>
Output:
4321
Explanation:
In the exercise above,
- The function "reverse_string()" takes a single parameter '$str1', representing the input string to be reversed.
- It calculates the length of the input string using 'strlen($str1)' and stores it in the variable '$n'.
- If the length of the string is 1, it means the string is already reversed, so it returns the string itself.
- Otherwise, it decrements the length of the string by 1 ($n--), and then recursively reverses the substring starting from the second character using 'substr($str1, 1, $n)', and concatenates the first character using 'substr($str1, 0, 1)'.
- This recursive process continues until the base case (string length equals 1) is reached, and the reversed string is gradually built up.
- Finally, the function is called with the argument '1234', and the reversed string is printed using "print_r()" function.
Flowchart :
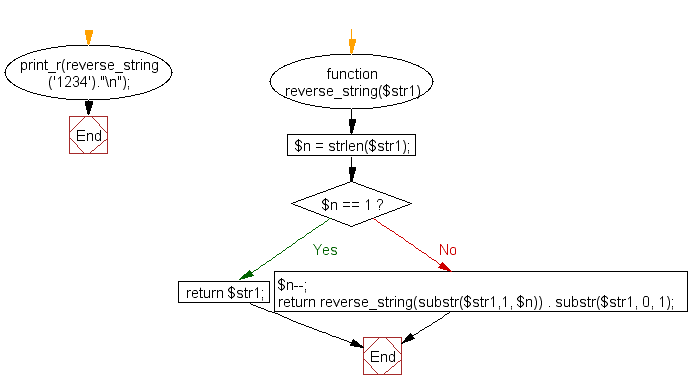
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a function to check a number is prime or not.
Next: Write a function to sort an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics