PHP function Exercises: Sort an array
4. Array Sorting
Write a PHP function to sort an array.
Visual Presentation:
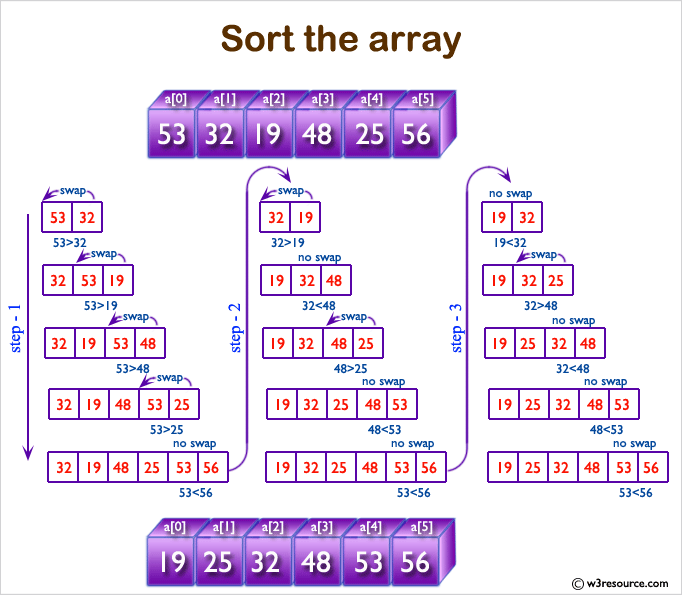
Sample Salution:
PHP Code:
<?php
// Function to sort an array in ascending order
function array_sort($a)
{
// Iterate through each element of the array
for ($x = 0; $x < count($a); ++$x)
{
// Assume the current element is the minimum
$min = $x;
// Iterate through the remaining elements of the array
for ($y = $x + 1; $y < count($a); ++$y)
{
// If a smaller element is found, update the minimum index
if ($a[$y] < $a[$min])
{
$temp = $a[$min];
$a[$min] = $a[$y];
$a[$y] = $temp;
}
}
}
// Return the sorted array
return $a;
}
// Input array
$a = array(51, 14, 1, 21, 'hj');
// Call the array_sort function with the input array and print the sorted array
print_r(array_sort($a));
?>
Output:
Array ( [0] => hj [1] => 1 [2] => 14 [3] => 21 [4] => 51 )
Explanation:
In the exercise above,
- The function "array_sort()" is defined, which takes an array '$a' as input.
- It iterates through each array element using a for loop, starting from the first element.
- Inside the outer loop, another inner loop is used to find the minimum element among the unsorted elements.
- The index of the minimum element is stored in the variable '$min'.
- If a smaller element is found in the inner loop, it swaps the positions of the current minimum element and the new minimum element.
- After completing the inner loop, the minimum element is placed in its correct position in the array.
- This process continues until the entire array is sorted.
- Finally, the sorted array is returned.
Flowchart :
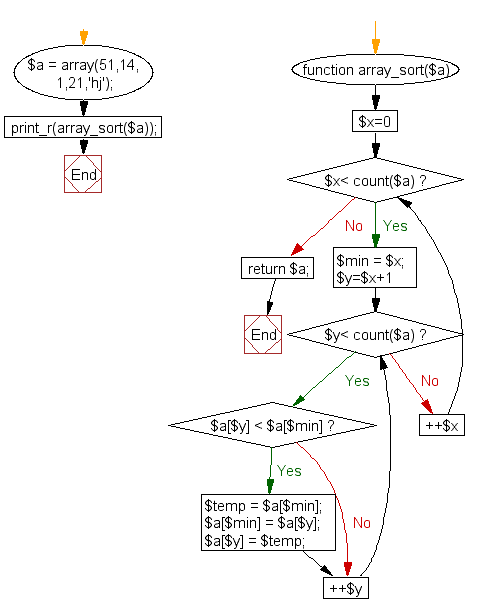
For more Practice: Solve these Related Problems:
- Write a PHP function to implement the bubble sort algorithm on an array of integers and output the number of passes it took to sort the array.
- Write a PHP script to sort an array of strings in natural case-insensitive order without using built-in sorting functions.
- Write a PHP function to perform a quicksort on an array and then compare its efficiency with merge sort on the same dataset.
- Write a PHP program that sorts an associative array by its values using a custom comparison function and preserves the keys.
Go to:
PREV : String Reversal.
NEXT : Check All Lowercase String.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.