PHP String Exercises : Generate simple random password from a specified string
PHP String: Exercise-9 with Solution
Write a PHP script to generate simple random password [do not use rand() function] from a given string.
Sample string: '1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZabcefghijklmnopqrstuvwxyz'
Note : Password length may be 6, 7, 8 etc.
Sample Solution:
PHP Code:
<?php
// Define a function named password_generate that takes the number of characters as input
function password_generate($chars)
{
// Define a string containing all possible characters for the password
$data = '1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZabcefghijklmnopqrstuvwxyz';
// Shuffle the characters in the string and extract a substring of length $chars
return substr(str_shuffle($data), 0, $chars);
}
// Call the password_generate function with 7 characters and echo the generated password
echo password_generate(7)."\n";
?>
Output:
89Qp3AK
Explanation:
In the exercise above,
- <?php: This tag indicates the beginning of a PHP script.
- Function password_generate($chars): This line defines a PHP function named "password_generate()" that takes one parameter '$chars', which represents the number of characters in the password to be generated.
- {: Opening curly brace indicates the beginning of the function body.
- $data = '1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZabcefghijklmnopqrstuvwxyz';: This line creates a string named '$data' containing a pool of characters from which the password will be generated. It includes digits (0-9), uppercase letters (A-Z), and lowercase letters (a-z).
- return substr(str_shuffle($data), 0, $chars);: This line generates a random password by performing the following steps:
- str_shuffle($data): Randomly shuffles the characters in the '$data' string.
- substr(...): Takes a substring of the shuffled characters, starting from index 0 and having a length of '$chars'. This ensures that the generated password has the specified number of characters.
- return ...;: Returns the generated password from the function.
- }: Closing curly brace indicates the end of the function body.
- echo password_generate(7)."\n";: This line calls the "password_generate()" function with an argument of 7, indicating that a password with 7 characters should be generated. It then echoes the generated password followed by a newline character.
- ?>: This tag indicates the end of the PHP script.
Flowchart :
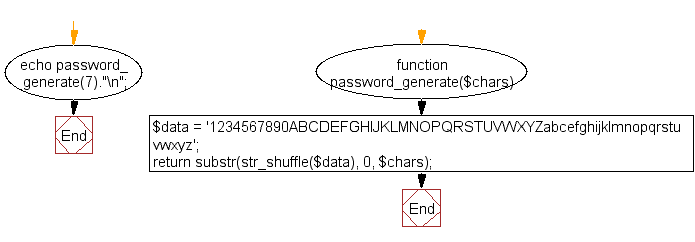
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to format values in currency style.
Next: Write a PHP script to replace the first 'the' of the following string with 'That'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics