Running asynchronous Python functions with different time delays
2. Multiple Async Functions with Delays
Write a Python program that creates three asynchronous functions and displays their respective names with different delays (1 second, 2 seconds, and 3 seconds).
Sample Solution:
Code:
import asyncio
async def display_name_with_delay(name, delay):
await asyncio.sleep(delay)
print(name)
async def main():
tasks = [
display_name_with_delay("Asyn. function-1", 1),
display_name_with_delay("Asyn. function-2", 2),
display_name_with_delay("Asyn. function-3", 3)
]
await asyncio.gather(*tasks)
# Run the event loop
asyncio.run(main())
Output:
Asyn. function-1 Asyn. function-2 Asyn. function-3
Explanation:
In the above exercise,
- "display_name_with_delay()" is an asynchronous function that takes a 'name' and a 'delay' as arguments. It uses await asyncio.sleep(delay) to introduce the specified delay, and then displays the given name.
- The main coroutine runs the three asynchronous functions concurrently using the asyncio.gather() function. It creates a list of tasks representing each call to print_name_with_delay.
- The asyncio.run(main()) line starts the event loop, which runs the concurrent tasks defined in the tasks list.
When you run this program, you'll see the names "Asyn. function-1", "Asyn. function-2", "Asyn. function-3" displayed on the console with delays of 1 second, 2 seconds, and 3 seconds respectively.
Flowchart:
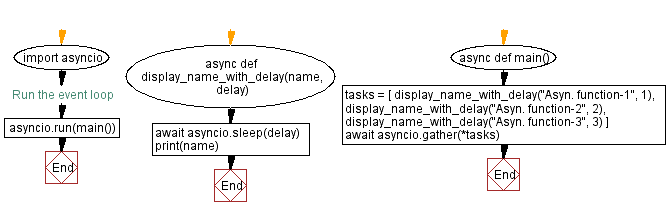
For more Practice: Solve these Related Problems:
- Write a Python program that creates three asynchronous functions, each printing its unique identifier after delays of 1, 2, and 3 seconds respectively, then schedules them concurrently.
- Write a Python script that defines three async functions which print different messages after 1, 2, and 3 seconds, and then gathers them with asyncio.gather to display the outputs in order of completion.
- Write a Python function that uses asyncio to run three tasks with varying sleep times (1s, 2s, 3s) and prints a summary when all tasks are finished.
- Write a Python program to implement three asynchronous functions that log their start and finish times after delays of 1, 2, and 3 seconds respectively, and then output the total execution time.
Go to:
Previous: Delaying Print Output with asyncio Coroutines in Python.
Next: Printing numbers with a delay using asyncio coroutines in Python.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.