Delaying Print Output with asyncio Coroutines in Python
1. Async Print with Delay
Write a Python program that creates an asynchronous function to print "Python Exercises!" with a two second delay.
Sample Solution:
Code:
import asyncio
async def print_delayed_message():
await asyncio.sleep(2) # Wait for 2 seconds
print("Python Exercises!")
# Create an event loop and run the asynchronous function
async def main():
await print_delayed_message()
# Run the event loop
asyncio.run(main())
Output:
Python Exercises!
Explanation:
In the above exercise, the "delayed_message()" function is defined as an asynchronous coroutine. It uses the await asyncio.sleep(2) statement to pause the execution of the coroutine for 2 seconds. Then, "Python Exercises!" is printed to the console.
The main coroutine runs the delayed_message coroutine. The asyncio.run(main()) line starts the event loop, which executes the asynchronous operations defined in the coroutines.
Flowchart:
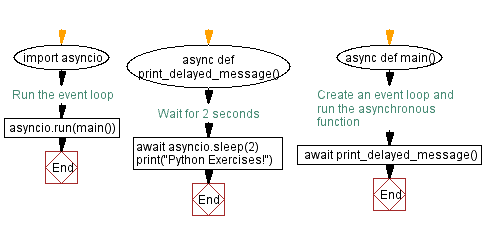
For more Practice: Solve these Related Problems:
- Write a Python program that defines an asynchronous function to print "Hello, Async World!" after a delay of 2 seconds using asyncio.sleep, and then call it from an event loop.
- Write a Python function that asynchronously prints "Delayed Message" after waiting for 2 seconds and then returns a confirmation string.
- Write a Python script that creates an async function which prints "Async in Action!" with a two-second delay, and then schedules this function multiple times concurrently.
- Write a Python program to implement an asynchronous function that prints a custom message after a 2-second delay and logs the time before and after the print.
Go to:
Previous: Python Asynchronous Exercises Home.
Next: Running asynchronous Python functions with different time delays.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.