Measuring total execution time of concurrent asynchronous tasks in Python
5. Asyncio Gather Tasks
Write a Python program that runs multiple asynchronous tasks concurrently using asyncio.gather() and measures the time taken.
Sample Solution:
Code:
import asyncio
import time
async def task1():
print("Task-1 started")
await asyncio.sleep(4)
print("Task-1 completed")
async def task2():
print("Task-2 started")
await asyncio.sleep(1)
print("Task-2 completed")
async def task3():
print("Task-3 started\n")
await asyncio.sleep(2)
print("Task-3 completed")
async def main():
start_time = time.time()
await asyncio.gather(task1(), task2(), task3())
end_time = time.time()
elapsed_time = end_time - start_time
print("\nAll tasks completed in {:.2f} seconds".format(elapsed_time))
# Run the event loop
asyncio.run(main())
Output:
Task-1 started Task-2 started Task-3 started Task-2 completed Task-3 completed Task-1 completed All tasks completed in 4.00 seconds
Explanation:
In the above exercise-
- Three asynchronous tasks (task1, task2, and task3) are defined. Each task simulates a different duration of work using await asyncio.sleep().
- The main coroutine starts by recording the start time using time.time().
- The asyncio.gather() function runs all tasks concurrently. It takes the tasks as arguments and runs them concurrently, waiting for all of them to complete.
- After the asyncio.gather() call, the end time is recorded using time.time(), and the elapsed time is calculated.
- The program prints a message indicating that all tasks have been completed and displays the elapsed time.
Flowchart:
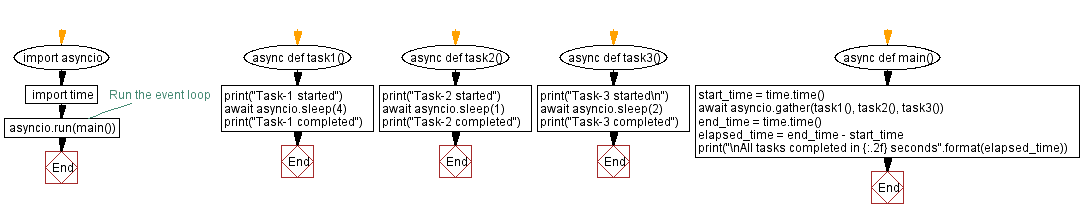
For more Practice: Solve these Related Problems:
- Write a Python program to run three asynchronous tasks concurrently using asyncio.gather() and print the total execution time once all tasks complete.
- Write a Python script to schedule multiple async functions with varying delays using asyncio.gather and then output the order in which they complete.
- Write a Python function that executes a list of asynchronous tasks concurrently with asyncio.gather, then returns a list of results along with the total elapsed time.
- Write a Python program to implement a set of concurrent tasks with asyncio.gather(), measure the time taken for execution, and print both the results and the elapsed time.
Go to:
Previous: Fetching data asynchronously from multiple URLs using Python async IO.
Next: Check list sorting.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.