Creating a Python decorator to convert function return value
Python Decorator: Exercise-3 with Solution
Write a Python program to create a decorator to convert the return value of a function to a specified data type.
Sample Solution:
Python Code:
def convert_to_data_type(data_type):
def decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return data_type(result)
return wrapper
return decorator
@convert_to_data_type(int)
def add_numbers(x, y):
return x + y
result = add_numbers(10, 20)
print("Result:", result, type(result))
@convert_to_data_type(str)
def concatenate_strings(x, y):
return x + y
result = concatenate_strings("Python", " Decorator")
print("Result:", result, type(result))
Sample Output:
Result: 30 <class 'int'> Result: Python Decorator <class 'str'>
Explanation:
In the above exercise -
- The "convert_to_data_type()" function takes a 'data_type' parameter, which specifies the desired data type for the return value.
- Inside the "convert_to_data_type function()", a decorator function is defined. This decorator takes the "func" function as an argument.
- Within the decorator, a nested function called a wrapper is defined. This function serves as a wrapper around the original function.
- Inside the wrapper function, the original function is called with the provided arguments (*args and **kwargs), and the return value is stored in the result variable.
- The 'result' is then converted to the specified 'data_type' using data_type(result).
- The converted value is returned from the wrapper function.
- The "decorator()" function is returned from the 'convert_to_data_type function', completing the decoration process.
- The @convert_to_data_type(int) decorator is applied to the add_numbers function, indicating that the return value should be converted to an integer.
- The '@convert_to_data_type(str)' decorator is applied to the concatenate_strings function, indicating that the return value should be converted to a string.
- The decorated functions are called with the provided arguments, and the converted results are printed along with their data types.
Upon running this code, you will see the converted results and their corresponding data types. For example, the add_numbers function returns the sum of two numbers, converted to an integer. In contrast, the concatenate_strings function concatenates two strings and converts the result to a string data type.
Flowchart:
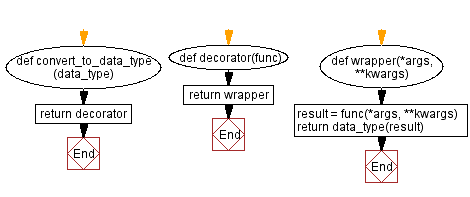
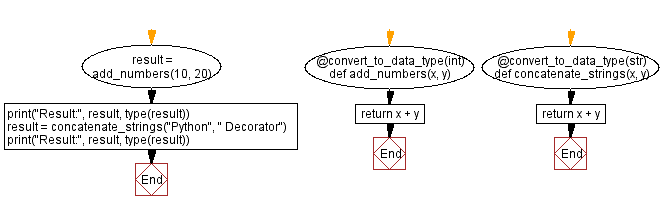
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Creating a Python decorator to measure function execution time.
Next: Implementing a Python decorator for function results caching.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics