Python user authentication using Boolean logic
Python Boolean Data Type: Exercise-10 with Solution
Write a Python program that checks a simple authentication system. The program verifies the provided username and password against predefined usernames and passwords by using boolean checks.
Sample Solution:
Code:
def user_authentication(username, password):
# Predefined usernames and passwords
predefined_users = {
"empdb1": "Jue@3$juy0",
"empdb2": "juRe34@$",
"admin": "koiUaq$&@ki"
}
if username in predefined_users and predefined_users[username] == password:
return True
else:
return False
def main():
try:
username = input("Input your userid: ")
password = input("Input your password: ")
if user_authentication(username, password):
print("Authentication has been successful. Welcome!", username)
else:
print("Authentication failed. Invalid username or password.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input your userid: empdb2 Input your password: juRe34@$ Authentication has been successful. Welcome! empdb
Input your userid: admin Input your password: kojUaq$&@ki Authentication failed. Invalid username or password.
In the above exercise the program prompts the user to input a username and password. Using the "user_authentication()" function, it then checks if the provided credentials match the predefined credentials. Finally it prints the appropriate message based on the authentication results.
Flowchart:
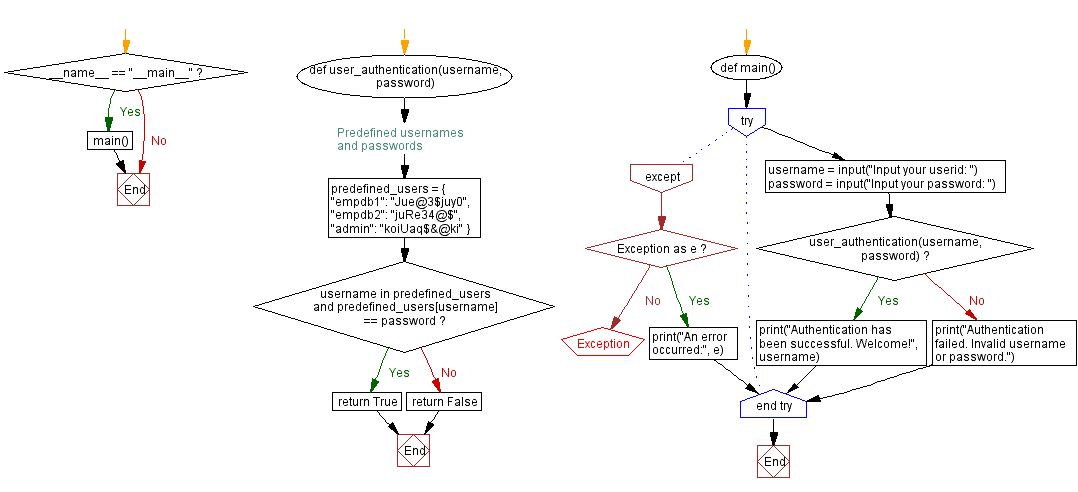
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics